A Comprehensive Guide to the ChaCha20 Algorithm
Discover the power of ChaCha20, a high-speed, secure stream cipher. This comprehensive guide explores its workings, advantages, and real-world applications in TLS/SSL, messaging apps, VPNs, and more. Learn how to implement ChaCha20 in Python and understand key security considerations.
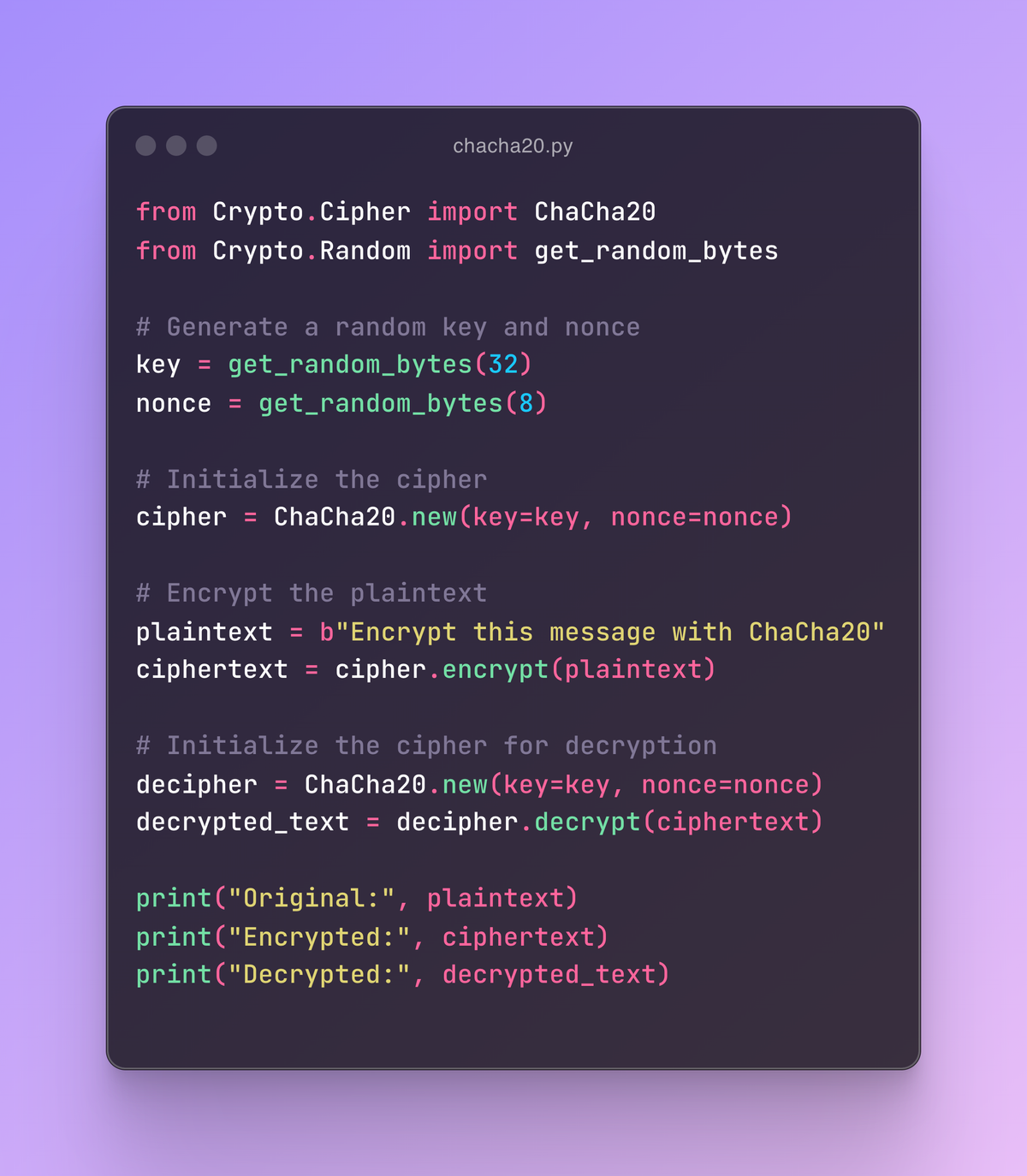
Introduction
The world of cybersecurity is constantly evolving, demanding robust and efficient cryptographic solutions. Among the many algorithms available, ChaCha20 has emerged as a popular choice for fast and secure encryption. This article will provide you with a comprehensive understanding of ChaCha20, including its inner workings, advantages, and real-world applications. By the end, you'll be equipped with the knowledge to leverage ChaCha20 in your own projects and understand its significance in modern cryptography.
Table of Contents
- Introduction
- What is the ChaCha20 Algorithm?
- Why Choose ChaCha20?
- How ChaCha20 Works
- Implementing ChaCha20: A Step-by-Step Tutorial
- Real-world Applications
- Security Considerations
- Conclusion
What is the ChaCha20 Algorithm?
ChaCha20 is a stream cipher designed by Daniel J. Bernstein. Stream ciphers, unlike block ciphers like AES, operate on data byte by byte, generating a keystream that is XORed with the plaintext to produce the ciphertext. ChaCha20 stands out for its high performance, strong security, and resistance to timing attacks, making it well-suited for various applications, including secure communication, data storage, and network encryption.
Why Choose ChaCha20?
Problems It Solves
- High-speed encryption and decryption: Crucial for real-time applications where performance is paramount.
- Strong security: Protects against known cryptanalytic attacks and offers robust security guarantees.
- Energy efficiency: Its lightweight design reduces computational overhead, ideal for resource-constrained devices.
Unique Insights
- Modern cryptographic needs: Addresses limitations of older ciphers, offering improved security and efficiency.
- Flexibility: Can be easily integrated into various systems without significant performance compromises.
Audience Interests
- Security professionals: Looking to enhance the security of their encryption protocols and systems.
- Developers: Seeking secure and efficient algorithms to implement in their applications.
- Students: Studying state-of-the-art cryptographic techniques and exploring their applications.
How ChaCha20 Works
Algorithm Overview
ChaCha20 uses a 512-bit state, represented as 16 32-bit words. This state is initialized with four constant words, eight key words (derived from the secret key), two counter words, and two nonce words (a unique value for each encryption). The heart of the algorithm lies in a series of quarter-round operations applied to this state.
Encryption Process
- Initialization:
- The initial state is set up with the provided key, nonce, and constants.
- Block Function:
- The state undergoes 20 rounds of 4 quarter-round operations, each round mixing the words in the state to create a highly scrambled output.
- Key Stream Generation:
- After the 20 rounds, the state is transformed into a key stream, which is essentially a sequence of pseudo-random bytes.
- Encryption:
- The plaintext is XORed with the key stream byte by byte, producing the ciphertext.
Decryption Process
Decryption is the reverse of encryption:
- Initialization: The initial state is set up using the same key and nonce as encryption.
- Block Function: 20 rounds of quarter-round operations are performed.
- Key Stream Generation: The key stream is generated from the final state.
- Decryption: The ciphertext is XORed with the key stream to recover the original plaintext.
Implementing ChaCha20: A Step-by-Step Tutorial
Setting Up Your Environment
Before implementing ChaCha20, make sure you have a suitable development environment:
- Install Python 3.x.
- Install the
pycryptodome
library, which provides convenient cryptographic functions for Python:pip install pycryptodome
Code Snippets
from Crypto.Cipher import ChaCha20
from Crypto.Random import get_random_bytes
# Generate a random key and nonce
key = get_random_bytes(32)
nonce = get_random_bytes(8)
# Initialize the cipher
cipher = ChaCha20.new(key=key, nonce=nonce)
# Encrypt the plaintext
plaintext = b"Encrypt this message with ChaCha20"
ciphertext = cipher.encrypt(plaintext)
# Initialize the cipher for decryption
decipher = ChaCha20.new(key=key, nonce=nonce)
decrypted_text = decipher.decrypt(ciphertext)
print("Original:", plaintext)
print("Encrypted:", ciphertext)
print("Decrypted:", decrypted_text)
This code snippet demonstrates a basic ChaCha20 implementation using pycryptodome
. It generates a random key and nonce, initializes the cipher, encrypts the plaintext, and decrypts it to verify the output.
Real-world Applications
ChaCha20 has gained significant traction in various real-world applications, including:
- TLS/SSL Protocols: Widely used in secure communication over the internet, protecting data transferred between clients and servers.
- Messaging Apps: Securing private messages sent between users, ensuring confidentiality and integrity.
- VPNs: Encrypting data traffic to protect user privacy and bypass censorship, ensuring secure access to online resources.
- Disk Encryption: Encrypting data stored on hard drives or other storage devices, safeguarding sensitive information from unauthorized access.
Security Considerations
While ChaCha20 is considered a strong and secure algorithm, it's essential to address potential security risks:
- Key Management: Securely generating, storing, and managing encryption keys is crucial. Compromised keys can compromise the entire encryption system.
- Nonce Reuse: Each encryption should use a unique nonce. Reusing nonces can lead to security vulnerabilities.
- Implementation Errors: Incorrect implementations can introduce weaknesses that attackers could exploit.
Conclusion
ChaCha20 stands out as a modern, fast, and secure encryption algorithm. Its high performance, strong security guarantees, and wide range of applications make it a valuable tool for developers and security professionals alike. By understanding its principles and best practices, you can effectively leverage ChaCha20 to protect your sensitive data in various contexts.