B-Tree File System Fundamentals: A Comprehensive Guide
Unlock the power of B-Tree file systems! Learn about their structure, benefits, and real-world applications. Discover how B-Trees revolutionize data management, from fast retrieval to efficient storage. Boost your system performance now! #FileSystems #DataStorage
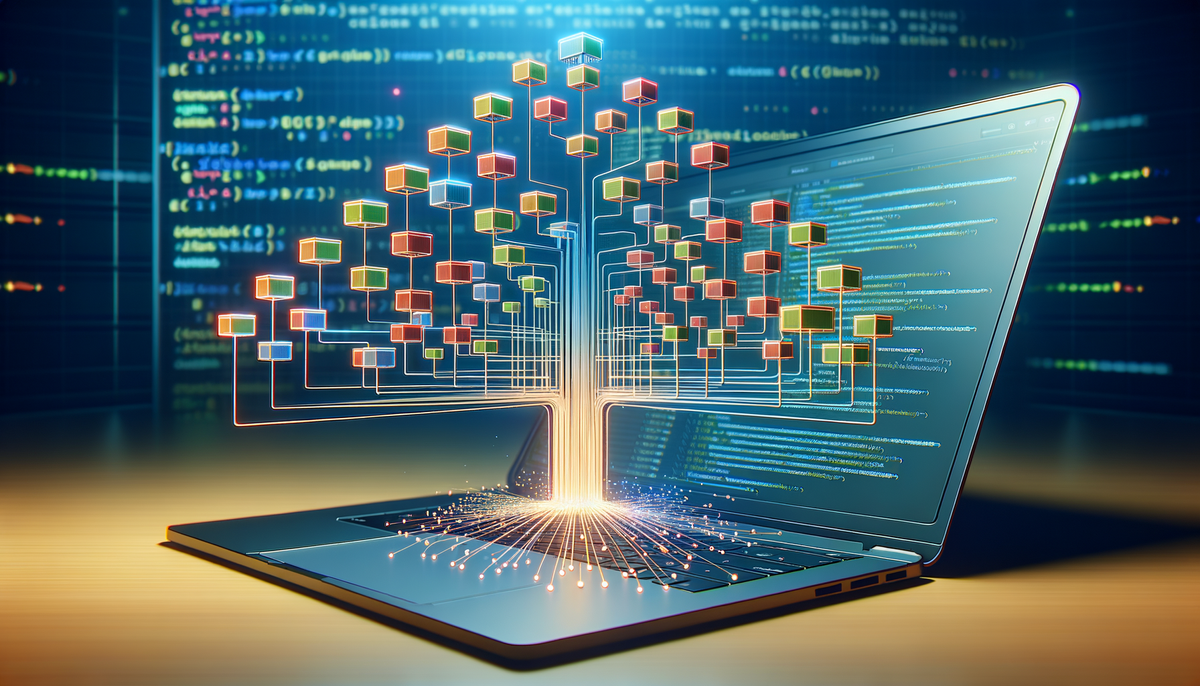
Introduction
File systems are the backbone of data storage and retrieval in modern computing. Among the various file system structures, B-Tree file systems stand out for their efficiency and reliability. This guide will walk you through the fundamentals of B-Tree file systems, exploring their structure, benefits, and real-world applications.
What is a B-Tree File System?
A B-Tree file system is a type of file system that uses B-Tree data structures to organize and manage files and directories. B-Trees are self-balancing tree data structures that maintain sorted data and allow for efficient insertions, deletions, and searches.
Key Features:
- Fast data retrieval
- Efficient storage utilization
- Scalability for large datasets
- Balanced structure for consistent performance
How B-Trees Work
B-Trees are designed to work well with storage systems that read and write large blocks of data. Here's a simple breakdown of how they function:
- Nodes contain multiple keys and children
- Keys are sorted within each node
- All leaf nodes are at the same depth
- Internal nodes store keys and pointers to child nodes
- Leaf nodes store keys and data or pointers to data
Example B-Tree Structure:
[10, 20]
/ | \
[5] [15] [25, 30]
Advantages of B-Tree File Systems
B-Tree file systems offer several benefits over traditional file systems:
- Faster search operations
- Efficient use of disk space
- Better performance for large directories
- Reduced fragmentation
- Improved crash recovery
Implementing a Basic B-Tree
Here's a simple Python implementation of a B-Tree node:
class BTreeNode:
def __init__(self, t, leaf=False):
self.t = t # Minimum degree
self.leaf = leaf
self.keys = []
self.children = []
def insert_non_full(self, k):
i = len(self.keys) - 1
if self.leaf:
self.keys.append(None)
while i >= 0 and k < self.keys[i]:
self.keys[i + 1] = self.keys[i]
i -= 1
self.keys[i + 1] = k
else:
while i >= 0 and k < self.keys[i]:
i -= 1
i += 1
if len(self.children[i].keys) == (2 * self.t - 1):
self.split_child(i, self.children[i])
if k > self.keys[i]:
i += 1
self.children[i].insert_non_full(k)
def split_child(self, i, y):
z = BTreeNode(y.t, y.leaf)
self.children.insert(i + 1, z)
self.keys.insert(i, y.keys[self.t - 1])
z.keys = y.keys[self.t:]
y.keys = y.keys[:self.t - 1]
if not y.leaf:
z.children = y.children[self.t:]
y.children = y.children[:self.t]
B-Tree File System Operations
B-Tree file systems perform various operations to manage data efficiently:
- Insertion: Adding new files or directories
- Deletion: Removing files or directories
- Search: Finding specific files or directories
- Traversal: Listing contents of directories
- Balancing: Maintaining the tree structure for optimal performance
Insertion Process:
- Find the appropriate leaf node
- Insert the key if there's space
- If the node is full, split it and propagate changes upward
Deletion Process:
- Locate the key to be deleted
- If in a leaf node, simply remove it
- If in an internal node, replace with predecessor or successor
- Rebalance the tree if necessary
Real-World Applications
B-Tree file systems are widely used in various applications:
- Databases (e.g., MySQL, PostgreSQL)
- File systems (e.g., HFS+, Btrfs)
- Search engines
- Geographic information systems
Comparison with Other File Systems
Let's compare B-Tree file systems with other common file systems:
Feature | B-Tree FS | FAT | NTFS | ext4 |
---|---|---|---|---|
Max file size | Very large | 4GB | 16TB | 16TB |
Performance on large datasets | Excellent | Poor | Good | Good |
Fragmentation resistance | High | Low | Medium | High |
Metadata efficiency | High | Low | Medium | High |
Best Practices for B-Tree File Systems
To get the most out of B-Tree file systems:
- Optimize node size for your storage medium
- Implement proper caching mechanisms
- Use appropriate indexing strategies
- Regularly perform maintenance and optimizations
- Implement robust backup and recovery procedures
Challenges and Limitations
While B-Tree file systems offer many advantages, they also have some challenges:
- Complexity in implementation
- Potential for performance degradation with frequent updates
- Higher memory requirements compared to simpler structures
Future of B-Tree File Systems
As data storage needs continue to grow, B-Tree file systems are evolving:
- Integration with solid-state drives (SSDs)
- Improved compression techniques
- Enhanced security features
- Better support for distributed systems
B-Tree Variants and Optimizations
Several variants of B-Trees have been developed to address specific use cases and performance requirements:
-
B+ Trees: Commonly used in databases and file systems, B+ Trees store all data in leaf nodes, making range queries more efficient.
-
B Trees*: These maintain nodes at least 2/3 full, reducing the frequency of node splits and merges.
-
Counted B-Trees: These include additional information about the number of items in subtrees, useful for operations like finding the nth item.
-
Lazy B-Trees: These delay splits and merges to improve performance in scenarios with frequent updates.
Implementing B-Tree File Systems
When implementing a B-Tree file system, consider the following aspects:
-
Block Size: Choose an appropriate block size based on the underlying storage medium.
-
Caching: Implement efficient caching mechanisms to reduce disk I/O.
-
Concurrency Control: Use appropriate locking mechanisms for multi-threaded access.
-
Error Handling: Implement robust error detection and recovery mechanisms.
-
Journaling: Consider implementing journaling for improved crash recovery.
Here's a simple example of how to implement a basic file operation using a B-Tree structure:
class BTreeFileSystem:
def __init__(self, t):
self.root = BTreeNode(t, leaf=True)
self.t = t
def create_file(self, filename, content):
key = self.hash_filename(filename)
if len(self.root.keys) == (2 * self.t - 1):
new_root = BTreeNode(self.t, leaf=False)
new_root.children.append(self.root)
new_root.split_child(0, self.root)
self.root = new_root
self.root.insert_non_full((key, content))
def read_file(self, filename):
key = self.hash_filename(filename)
result = self.search(self.root, key)
return result[1] if result else None
def hash_filename(self, filename):
return sum(ord(c) for c in filename)
def search(self, node, key):
i = 0
while i < len(node.keys) and key > node.keys[i][0]:
i += 1
if i < len(node.keys) and key == node.keys[i][0]:
return node.keys[i]
elif node.leaf:
return None
else:
return self.search(node.children[i], key)
Performance Tuning
To optimize B-Tree file system performance:
-
Adjust Node Size: Find the optimal node size for your specific use case.
-
Implement Buffer Pool: Use a buffer pool to cache frequently accessed nodes.
-
Optimize I/O Operations: Minimize disk reads and writes by batching operations.
-
Use Compression: Implement data compression to reduce storage requirements and I/O.
-
Regular Maintenance: Perform periodic defragmentation and rebalancing.
Security Considerations
When implementing B-Tree file systems, consider these security aspects:
-
Access Control: Implement robust access control mechanisms.
-
Encryption: Use encryption for sensitive data.
-
Auditing: Implement logging and auditing features.
-
Integrity Checks: Regularly perform integrity checks on the file system structure.
Conclusion
B-Tree file systems provide a powerful and efficient solution for managing large amounts of data. By understanding their structure and operations, developers and system administrators can leverage their benefits to build more robust and scalable applications.
Whether you're working on database management systems, developing file systems, or simply wanting to understand the technology behind your storage solutions, B-Tree file systems offer a wealth of knowledge and practical applications.
By mastering B-Tree file systems, you'll be better equipped to tackle complex data storage challenges and optimize your systems for peak performance. The principles learned here can be applied to various domains, from database design to operating system development, making B-Tree file systems a valuable tool in any developer's toolkit.