Building a CLI Application with Go: A Practical Example
Discover how to build a Go-powered CLI app with this step-by-step guide! Learn about Go's speed, simplicity, and concurrency, and create a versatile greeting app. Perfect for developers seeking to enhance their toolkits with custom command-line solutions.
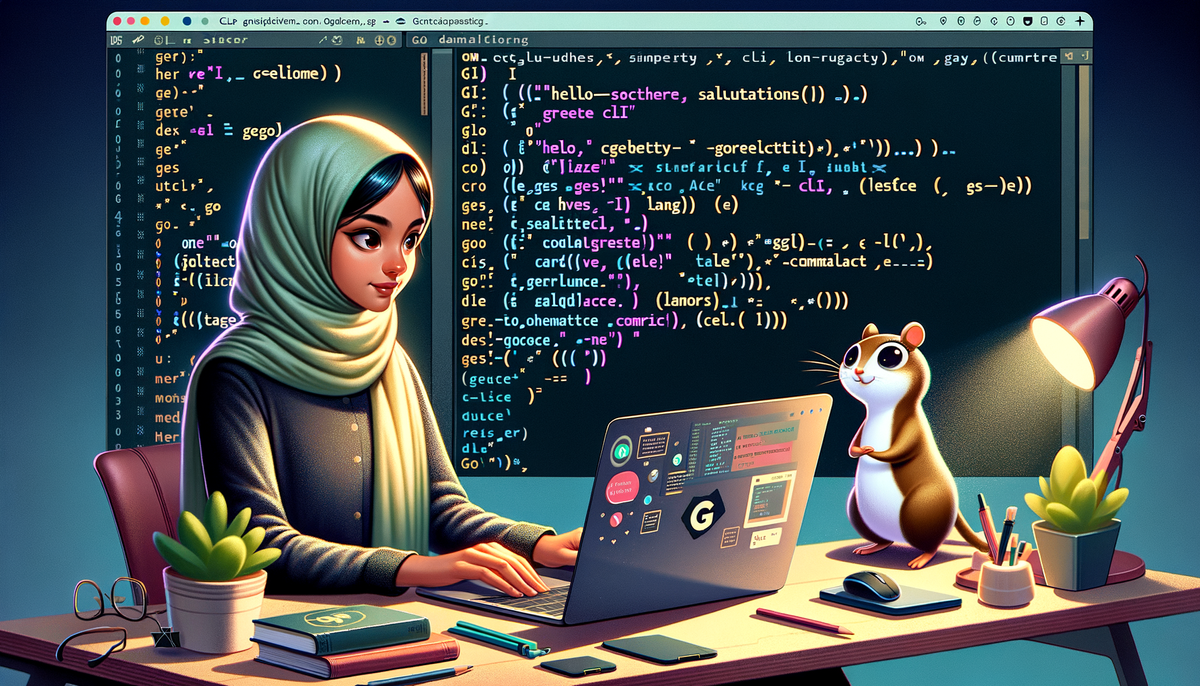
Command Line Interface (CLI) applications allow us to interact with computers using typed commands. They are efficient, fast, and an essential tool for developers. This guide will walk you through creating a simple CLI application using Go, a language loved for its speed and simplicity.
Why Build a CLI Application with Go?
Go, also known as Golang, is a perfect choice for building CLI applications because of its features:
- Speed: It's a compiled language, so it runs very fast.
- Simplicity: It features a straightforward syntax that's easy to understand.
- Concurrency: Go makes it easy to write programs that can do many tasks at once.
- Portability: Programs written in Go can run on different operating systems.
These qualities make Go ideal for CLI applications that need to work efficiently across various platforms.
Getting Started
Before jumping in, ensure you have Go installed. To check, open your terminal and type:
go version
If Go is not installed, visit Go's official website and follow the setup instructions.
Step 1: Set Up Your Project
Let's start by creating a new project directory for our CLI application.
- Open your terminal.
- Create a new directory named
greetcli
. - Move into this directory.
mkdir greetcli
cd greetcli
Next, create a Go file for our project called main.go
.
touch main.go
Step 2: Writing the Basic CLI
Open main.go
in a text editor and start by adding the package declaration and import statements:
package main
import (
"fmt"
"os"
)
The os
package helps manage system-level operations like reading command-line arguments. The fmt
package is used for formatted input and output.
Handling Command-Line Arguments
CLI applications expect input from users, typically via arguments. Let's handle that:
func main() {
if len(os.Args) < 2 {
fmt.Println("Please provide a name")
return
}
name := os.Args[1]
greetUser(name)
}
func greetUser(name string) {
message := fmt.Sprintf("Hello, %s!", name)
fmt.Println(message)
}
Explanation:
os.Args
gives us access to command-line arguments.- If no name is given, the application requests one.
greetUser
is a helper function that prints out a friendly greeting.
Running the CLI Application
Navigate to your greetcli
directory in the terminal. Use this command to run our app:
go run main.go Alice
You should see:
Hello, Alice!
Step 3: Adding More Features
Let's enhance our application with additional functionalities, like greeting styles and language options.
Adding Options
Update main.go
to include more options:
package main
import (
"fmt"
"os"
"strings"
)
func main() {
if len(os.Args) < 2 {
fmt.Println("Please provide a name. Usage: greetcli <name> --salutation=<type> --lang=<language>")
return
}
name := os.Args[1]
salutation := "Hello"
language := "en"
for _, arg := range os.Args[2:] {
if strings.HasPrefix(arg, "--salutation=") {
parts := strings.Split(arg, "=")
if len(parts) == 2 {
salutation = parts[1]
}
}
if strings.HasPrefix(arg, "--lang=") {
parts := strings.Split(arg, "=")
if len(parts) == 2 {
if parts[1] == "es" {
language = "es"
name = resolveSpanishName(name)
}
}
}
}
greetUser(name, salutation, language)
}
func greetUser(name, salutation, language string) {
message := fmt.Sprintf("%s, %s!", salutation, name)
fmt.Println(message)
}
func resolveSpanishName(name string) string {
if name == "Alice" {
return "Alicia"
}
return name
}
Running the Enhanced CLI
Try these commands:
go run main.go Alice
go run main.go Alice --salutation=hi
go run main.go Alice --lang=es
Explanation
- We loop through
os.Args
to parse options like--salutation
and--lang
. - The
resolveSpanishName
function changes names to a different language if applicable.
Step 4: Making It Executable
To turn your Go script into an executable file, build it using:
go build -o greetcli
This creates an executable called greetcli
. You can run it with:
./greetcli Alice --salutation=hi --lang=es
Conclusion
Congratulations! You've successfully built a functional CLI application using Go. Your app can now greet users with customizable greetings and language options. This project demonstrates Go's simplicity and efficiency in creating command-line tools. From here, consider adding advanced features like argument validation, error handling, or even reading from and writing to files.
Building CLI applications enriches your developer toolkit and opens up new possibilities for automation and scripting. Enjoy coding with Go!