C# vs Golang: Which Language Should You Choose?
C# vs Golang: Which programming language is right for you? This article compares these popular languages, highlighting their strengths, weaknesses, and use cases. Learn about their key features, performance, concurrency, and ecosystems to make the best choice for your project.
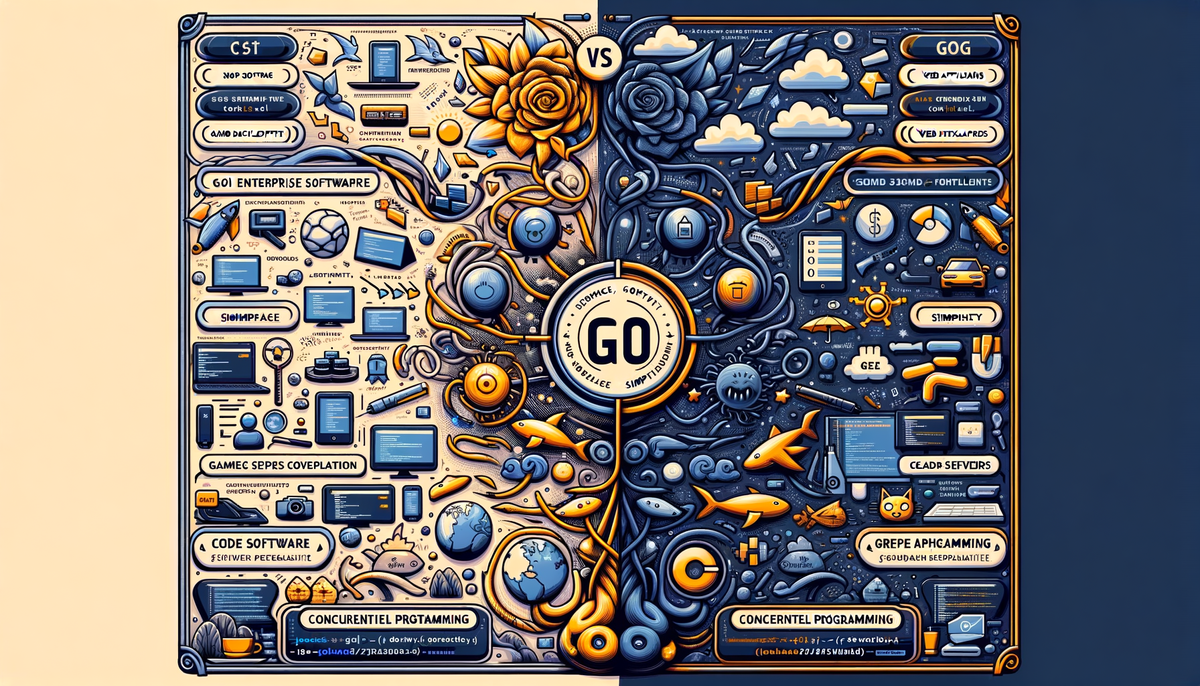
Choosing the right programming language can be tough. There are so many options, each with its own strengths and weaknesses. Today, we'll compare two popular languages: C# and Golang (Go). By the end, you'll know which one is right for your project.
Introduction
C# and Golang are popular choices for building a wide range of applications. C# is a mature language often used for enterprise software, while Golang is known for its speed and concurrency. Let's dive deeper into each language and how they stack up against each other.
What is C#?
C# is a language developed by Microsoft. It's a powerful language that can be used to build all sorts of things, like websites, apps for your phone, and even games. C# is a bit like a grown-up language; it's got lots of tools and features, and it's been around for a long time.
Key Features
- Object-Oriented: C# uses a style of programming called object-oriented programming (OOP). It's like building with LEGOs, where you create objects that interact with each other.
- Lots of Libraries: C# has a big library of pre-made tools that can help you do things quickly. It's like having a toolbox full of hammers, screwdrivers, and wrenches.
- Works on Different Computers: C# can run on different types of computers, like Windows, Mac, and Linux.
- Strict Rules: C# has strict rules about how you write code. This helps to avoid mistakes and makes sure your code works correctly.
Example: A Simple C# Program
Here's a simple C# program that creates a car object:
using System;
public class Car
{
public string Model { get; set; }
public Car(string model)
{
Model = model;
}
public void Drive()
{
Console.WriteLine($"{Model} is driving.");
}
}
class Program
{
static void Main()
{
Car myCar = new Car("Tesla");
myCar.Drive();
}
}
This program creates a Car
object with the model "Tesla" and then calls the Drive()
method to print a message.
What is Golang?
Golang, often called Go, is a language created by Google. It's known for being simple, fast, and good at handling lots of things at once. Imagine Go as a super-fast car that can easily handle any road, even a busy highway!
Key Features
- Easy to Learn: Go is designed to be simple and easy to understand, even if you're new to programming.
- Great for Many Tasks at Once: Go is really good at handling many tasks at the same time, making it perfect for web servers and other applications that need to do lots of things quickly.
- Fast and Efficient: Go is known for being fast and using your computer's resources wisely.
- Built-in Cleaning: Go has a built-in system that cleans up memory automatically, so you don't have to worry about it.
Example: A Simple Go Program
Here's a simple Go program that also creates a car object:
package main
import "fmt"
type Car struct {
Model string
}
func (c Car) Drive() {
fmt.Printf("%s is driving.\n", c.Model)
}
func main() {
myCar := Car{Model: "Tesla"}
myCar.Drive()
}
This program creates a Car
object with the model "Tesla" and then calls the Drive()
method to print a message.
Syntax Comparison
Let's see how the languages compare when writing a simple "Hello World" program:
Hello World in C#
using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello, World!");
}
}
Hello World in Go
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Go's syntax is simpler and more concise, which is one of its strengths.
Performance
Performance is crucial for many applications. Generally, Go applications are faster and use less memory than C# applications. This is because Go uses lightweight routines and manages memory efficiently. However, C# can be equally performant for high-performance enterprise applications that leverage .NET optimizations.
Benchmark Example
Let's look at a simple example where we add up a billion numbers:
C#
using System;
using System.Diagnostics;
class Program
{
static void Main()
{
long sum = 0;
Stopwatch stopwatch = Stopwatch.StartNew();
for (int i = 0; i < 1_000_000_000; i++)
{
sum += i;
}
stopwatch.Stop();
Console.WriteLine($"C# Sum: {sum} Time: {stopwatch.ElapsedMilliseconds}ms");
}
}
Go
package main
import (
"fmt"
"time"
)
func main() {
var sum int64
start := time.Now()
for i := int64(0); i < 1_000_000_000; i++ {
sum += i
}
elapsed := time.Since(start)
fmt.Printf("Go Sum: %d Time: %dms\n", sum, elapsed.Milliseconds())
}
These examples show how each language handles loops and resource allocation.
Concurrency
Concurrency is about handling multiple tasks at the same time. Golang shines in this area with its built-in support for concurrent programming.
Concurrency in C#
using System;
using System.Threading.Tasks;
class Program
{
static async Task Main()
{
await Task.Run(() => Console.WriteLine("Running asynchronously in C#"));
}
}
Concurrency in Go
package main
import "fmt"
import "time"
func main() {
go func() {
fmt.Println("Running concurrently in Go")
}()
time.Sleep(time.Second) // Give goroutine time to complete
}
Concurrency is simpler and more natural in Go than in C#.
Ecosystem and Libraries
C#
- Massive Library Collection: C# has a huge collection of pre-made tools and libraries for almost anything you can imagine.
- Mature Framework: C#'s framework has been around for a long time, making it very stable and reliable.
- Powerful Development Environment: Visual Studio is a powerful tool specifically designed for C# development.
Golang
- Growing Library Collection: Go has a good collection of libraries, especially for building web servers and networking applications.
- Fast and Simple Development: Go's simplicity and fast compilation make it a popular choice for many developers.
- Easy Package Management: Go makes it easy to manage and use third-party libraries.
Use Cases
C#
- Enterprise Applications: C# is a great choice for large, complex software projects, often used by big companies.
- Game Development: C# is widely used in game development, especially with the Unity engine.
- Web Services: C#'s ASP.NET Core framework is powerful for building web applications.
Golang
- Web Servers: Go is a great choice for building fast and efficient web servers.
- Cloud Services: Many cloud-based applications use Go due to its speed and concurrency.
- Command-Line Tools: Go's simplicity and performance make it ideal for building tools that run from the command line.
Conclusion
Both C# and Golang are excellent choices for different situations. C# is the go-to language for building robust enterprise applications and games, while Go excels in building high-performance, concurrent applications.
The best language for you depends on what you're building and your personal preferences. Consider the factors discussed in this article and choose the language that aligns with your project's needs and your development style. Happy coding!