Choosing Between gRPC and GraphQL: A Practical Guide
Struggling to choose between gRPC and GraphQL? This guide breaks down key factors like performance, flexibility, and use cases to help you decide. Learn about each technology's strengths, see real-world examples, and get practical tips for making the right choice for your project.
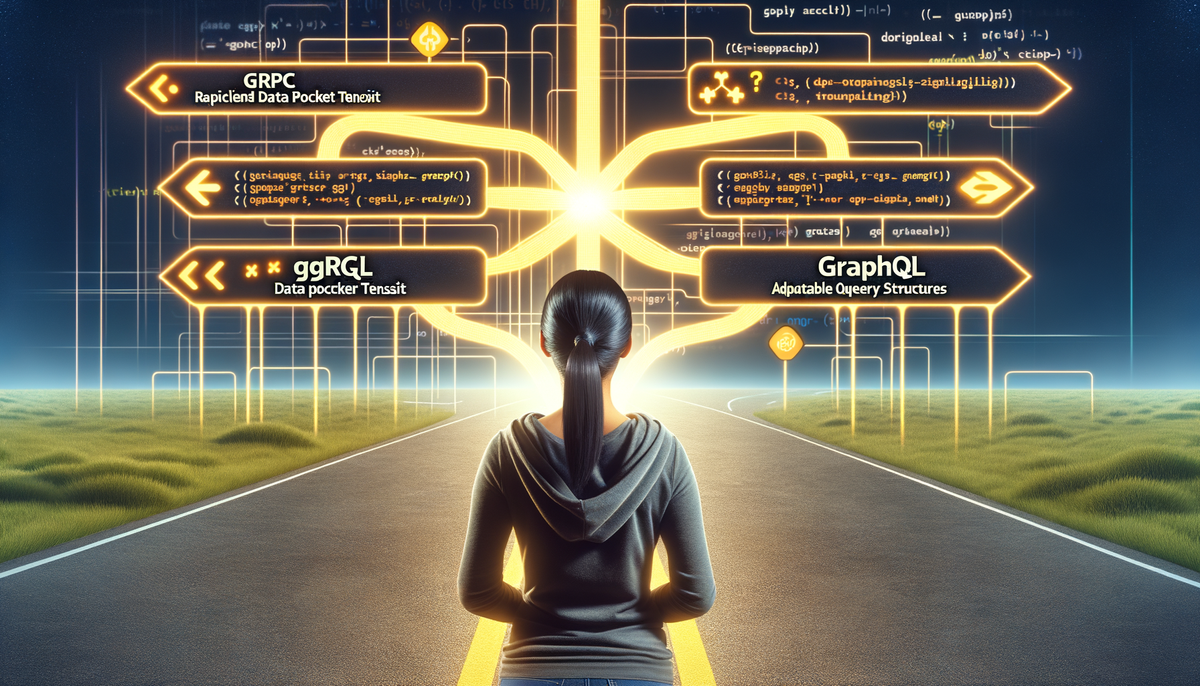
Are you struggling to decide between gRPC and GraphQL for your next project? You're not alone! Many developers face this challenge. In this comprehensive guide, we'll break down the key factors to help you make the right choice. We'll explore what these technologies are, how they work, and when to use each one.
What are gRPC and GraphQL?
Before we dive into the details, let's start with a simple explanation of these technologies:
- gRPC: A fast, open-source framework developed by Google for remote procedure calls (RPC).
- GraphQL: A query language and runtime for APIs, created by Facebook.
Both aim to improve communication between clients and servers, but they do it in different ways.
Key Factors to Consider
1. Performance
gRPC is known for its speed:
- Uses Protocol Buffers for efficient data serialization
- Supports HTTP/2 for faster, multiplexed connections
- Excellent for microservices and inter-service communication
GraphQL, while not as fast as gRPC, offers its own performance benefits:
- Reduces network traffic by allowing clients to request only the data they need
- Can aggregate data from multiple sources in a single request
Example:
// gRPC example
message User {
string id = 1;
string name = 2;
int32 age = 3;
}
service UserService {
rpc GetUser(UserId) returns (User) {}
}
# GraphQL example
type User {
id: ID!
name: String!
age: Int
}
type Query {
user(id: ID!): User
}
2. Flexibility
GraphQL offers more flexibility:
- Allows clients to request exactly the data they need
- Enables easy API evolution without versioning
- Great for front-end teams who need data in various shapes
gRPC is less flexible but more structured:
- Requires predefined service definitions
- Changes to the API often require updates to both client and server
3. Learning Curve
GraphQL is generally easier to learn:
- Uses a query language that resembles JSON
- More intuitive for developers familiar with REST
gRPC has a steeper learning curve:
- Requires understanding of Protocol Buffers
- Different paradigm compared to REST APIs
4. Tooling and Ecosystem
Both have robust ecosystems, but with different strengths:
- GraphQL has more tools for front-end development and API exploration (e.g., GraphiQL)
- gRPC excels in code generation and is favored in microservices architectures
5. Use Cases
gRPC shines in:
- Microservices architectures
- Systems requiring high-performance, low-latency communication
- Scenarios needing strong type checking and code generation
GraphQL excels in:
- Mobile and web applications requiring flexible data fetching
- APIs serving multiple client types with different data needs
- Situations where reducing over-fetching and under-fetching of data is crucial
6. Security
- gRPC: Built-in support for SSL/TLS, authentication, and encryption
- GraphQL: Requires additional setup for security measures, but offers fine-grained access control
7. Scalability
- gRPC: Excellent for scaling microservices architectures
- GraphQL: Good for scaling complex data requirements, but may require additional caching strategies
Making the Decision: A Simple Guide
To help you choose, consider these questions:
- Is raw performance the top priority?
- If yes, lean towards gRPC
- Do you need flexible data querying?
- If yes, GraphQL might be better
- Are you working on a microservices architecture?
- gRPC could be a good fit
- Is your team more familiar with REST-like APIs?
- GraphQL might be easier to adopt
- Do you need strong typing and automatic code generation?
- gRPC is excellent for this
- Are you building a public-facing API?
- GraphQL offers more flexibility to API consumers
Real-World Examples
Let's explore some practical examples to see how these technologies are used in real-life scenarios:
gRPC Example: IoT Device Management System
Imagine you're building an IoT device management system. Here's how you might define a service using gRPC:
syntax = "proto3";
service DeviceManagement {
rpc RegisterDevice (Device) returns (RegistrationResponse) {}
rpc UpdateFirmware (FirmwareUpdate) returns (UpdateStatus) {}
rpc MonitorDevice (DeviceId) returns (stream DeviceStatus) {}
}
message Device {
string id = 1;
string type = 2;
string firmware_version = 3;
}
message RegistrationResponse {
bool success = 1;
string message = 2;
}
message FirmwareUpdate {
string device_id = 1;
string new_version = 2;
bytes firmware_data = 3;
}
message UpdateStatus {
bool success = 1;
string message = 2;
}
message DeviceId {
string id = 1;
}
message DeviceStatus {
string id = 1;
double battery_level = 2;
bool online = 3;
repeated string active_sensors = 4;
}
This setup allows for efficient communication between IoT devices and the management system, with support for streaming real-time device status updates.
GraphQL Example: E-commerce Platform
Now, let's consider an e-commerce platform. Here's how you might structure a GraphQL schema:
type Product {
id: ID!
name: String!
description: String
price: Float!
category: Category!
inventory: Int!
reviews: [Review!]!
}
type Category {
id: ID!
name: String!
products: [Product!]!
}
type Review {
id: ID!
rating: Int!
comment: String
user: User!
}
type User {
id: ID!
name: String!
email: String!
orders: [Order!]!
}
type Order {
id: ID!
user: User!
items: [OrderItem!]!
total: Float!
status: OrderStatus!
}
type OrderItem {
product: Product!
quantity: Int!
}
enum OrderStatus {
PENDING
PROCESSING
SHIPPED
DELIVERED
}
type Query {
product(id: ID!): Product
products(category: ID, limit: Int): [Product!]!
categories: [Category!]!
user(id: ID!): User
order(id: ID!): Order
}
type Mutation {
createProduct(input: ProductInput!): Product!
updateProduct(id: ID!, input: ProductInput!): Product!
createOrder(input: OrderInput!): Order!
updateOrderStatus(id: ID!, status: OrderStatus!): Order!
}
input ProductInput {
name: String!
description: String
price: Float!
categoryId: ID!
inventory: Int!
}
input OrderInput {
userId: ID!
items: [OrderItemInput!]!
}
input OrderItemInput {
productId: ID!
quantity: Int!
}
This schema allows clients to request exactly the data they need, which is beneficial for both mobile apps with limited bandwidth and web applications requiring different data shapes for various views.
Conclusion: Making the Right Choice
Choosing between gRPC and GraphQL depends on your specific project requirements:
-
Choose gRPC if:
- You're building a microservices architecture
- Performance is critical
- You need strong typing and code generation
- You're working on systems with well-defined, stable interfaces
-
Choose GraphQL if:
- You're developing APIs for web and mobile apps with diverse data needs
- You need flexible data querying capabilities
- You want to reduce over-fetching and under-fetching of data
- Your API needs to evolve quickly without versioning
Remember, it's not always an either-or decision. Some projects successfully use both technologies where they fit best. The key is to understand your project's needs and choose the tool that aligns with your goals.
By considering factors like performance, flexibility, learning curve, available tooling, and specific use cases, you can make an informed decision that will set your project up for success. Happy coding, and may your APIs be ever efficient and developer-friendly!