Cookies vs. Tokens: Security, Performance, and Scalability
Choosing between cookies and tokens for user authentication? This article explores the pros and cons of each, examining security, performance, and scalability to help you decide the best fit for your website.
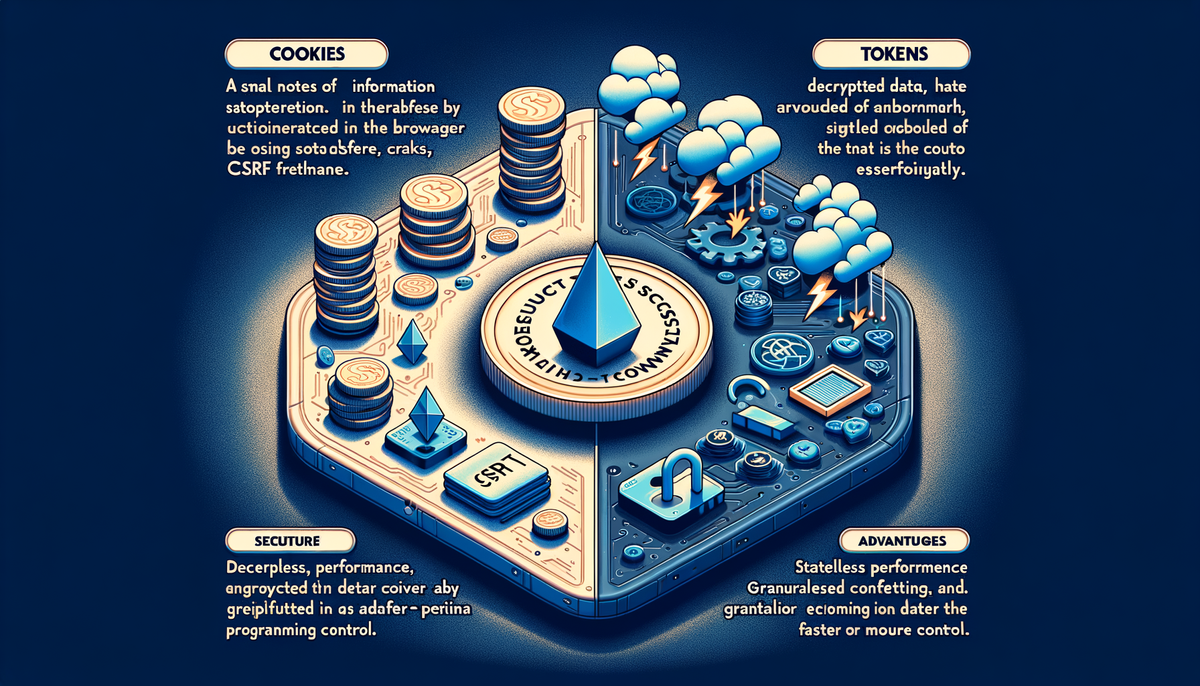
Introduction
When building websites, we need a way to keep track of who's logged in. Two popular methods for this are cookies and tokens. Cookies act like little notes your computer keeps about websites, while tokens are like secret codes for websites. Both have their advantages and disadvantages, so choosing the right one depends on what your website needs.
Cookies
What are Cookies?
Cookies are small pieces of information that websites store on your computer. They help the website remember things about you, like your login information or shopping cart items.
Cookies: Pros
- Easy to Use: Browsers handle cookies automatically, simplifying the development.
- Secure: You can tell browsers to only send cookies over secure connections (HTTPS) and stop JavaScript from reading them.
Cookies: Cons
- Vulnerability: If someone can steal your cookies, they can pretend to be you on the website.
- CSRF Attacks: Some tricks can force your browser to send cookies to other websites, even if you didn't want it to.
Tokens
What are Tokens?
Tokens are like secret codes that websites use to identify you. They are often stored in your web browser, so the website can check them when you visit.
Tokens: Pros
- Stateless: Websites don't have to store information about each user, which helps them work faster when many people use them.
- Granular Control: Tokens can be used to give you different permissions on a website.
Tokens: Cons
- Storage Issues: If someone can get access to your browser, they might be able to steal your tokens.
- Revoking Tokens: Removing tokens can be tricky, especially if they are valid for a long time.
Performance
Cookies: Performance
- Pros: Cookies are sent automatically with every request, so you don't need extra code to use them.
- Cons: Cookies can slow things down, especially if they are large.
Tokens: Performance
- Pros: Tokens are only sent when needed, so they can make things faster.
- Cons: You need extra code to handle tokens, which can make things more complex.
Scalability
Cookies: Scalability
- Pros: Cookies are easy to use for small websites.
- Cons: Websites that have lots of users might need extra servers to store all the cookie data.
Tokens: Scalability
- Pros: Tokens don't need extra servers to store data, which makes them good for websites with many users.
- Cons: Keeping tokens safe and revoking them can be tricky on larger websites.
Example: Building a Simple Token System
Here's how to create a simple token system using JavaScript and Node.js:
const express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
const secret = 'your_secret_key'; // Keep this safe!
// This function creates a token for a user
function createToken(user) {
const payload = { id: user.id, username: user.username }; // Information to include in the token
const token = jwt.sign(payload, secret); // Create the token
return token;
}
// This function checks if a token is valid
function verifyToken(token) {
try {
const decoded = jwt.verify(token, secret);
return decoded;
} catch (error) {
return false;
}
}
app.post('/login', (req, res) => {
const { username, password } = req.body;
// Check if the user exists and the password matches
if (username === 'test' && password === 'test') {
// Generate a token for the user
const token = createToken({ id: 1, username: 'test' });
res.json({ token });
} else {
res.status(401).send('Invalid credentials');
}
});
// This route requires a valid token
app.get('/protected', (req, res) => {
const token = req.headers.authorization; // Get the token from the request header
if (token) {
const decoded = verifyToken(token);
if (decoded) {
res.json({ message: 'You are authorized!', user: decoded });
} else {
res.status(401).send('Invalid token');
}
} else {
res.status(401).send('No token provided');
}
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
This code sets up a simple website with a login route and a protected route. The login route generates a token if the user provides correct credentials, and the protected route verifies the token before allowing access.
Conclusion
When deciding between cookies and tokens, consider the security, performance, and scalability needs of your website. Cookies are simpler but can have security problems, while tokens are more flexible but require careful management. No matter which method you choose, ensure to prioritize security and build your website to handle future growth.