Creating Custom Bash Functions: Automate Your Workflow with Scripting Power
Boost your command-line efficiency with custom Bash functions! Learn how to create shortcuts, automate tasks, and streamline your workflow with this beginner-friendly guide. Discover the power of Bash scripting and make your work easier and faster.
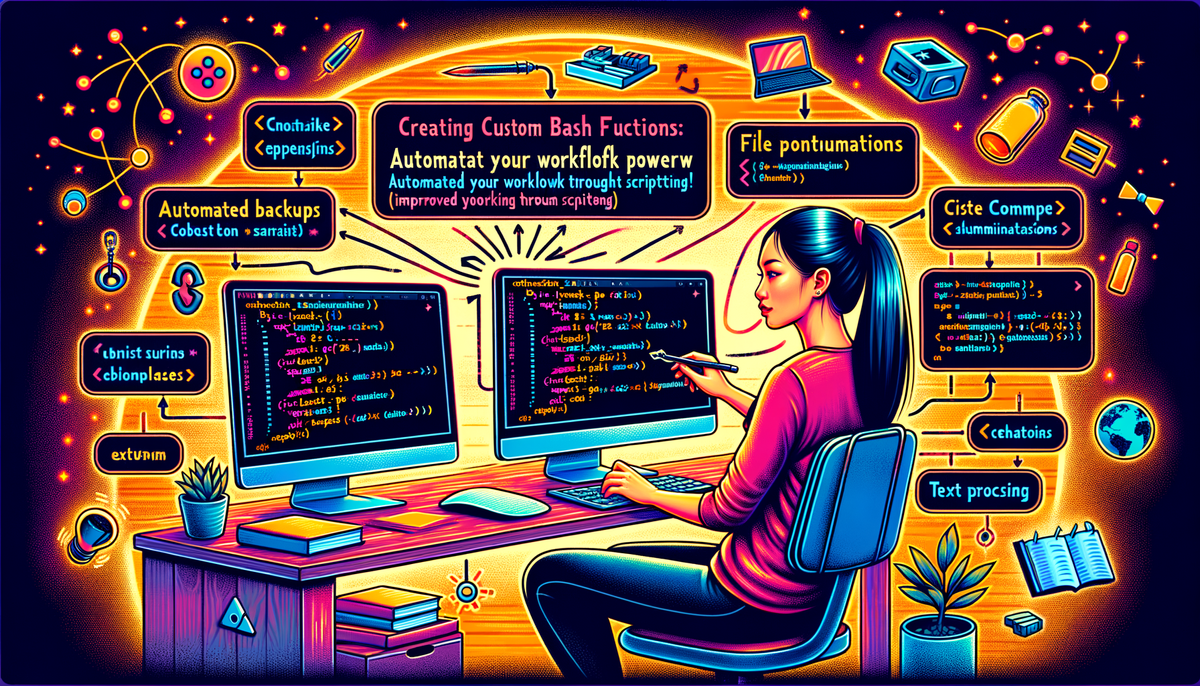
Bash is a powerful shell used by many developers and system administrators. A shell like Bash acts as an intermediary, taking commands from you and sending them to the operating system to be carried out. One of the cool things about Bash is that you can create your own custom functions. Imagine having a group of commands that you use often; you can bundle them together and run them with a single, easy-to-remember command! This article will guide you through creating and using your own custom Bash functions.
Why Use Custom Bash Functions?
Think of custom Bash functions as shortcuts for your everyday work in the command line. They offer a bunch of advantages:
- Less Typing, More Doing: Imagine repeating the same long series of commands over and over. With a custom function, you only need to type a short, easy-to-remember name.
- Automate Your Tasks: Tired of doing the same thing repeatedly? Bash functions let you automate those tasks so you can focus on more interesting things.
- Easy to Read, Easy to Understand: When you have a complex set of commands, it's hard to remember them all. A function helps you organize those commands into a single, clear command that's easier to understand.
Here are some cool things you can do with Bash functions:
- Automate backups: Keep your important files safe by creating a function to automatically back them up.
- Create custom shortcuts: Make your work faster by creating shortcut commands for tasks you do often.
- Process and manipulate text files: Bash functions can help you extract information from text files or modify their content.
Getting Started with Bash Functions: The Basics
Before we dive into examples, let's learn the basic structure of defining a Bash function.
The Function Formula
function_name() {
# This is where you put your commands!
}
Breaking it down:
function_name
: This is the name you will use to call your function. It's like giving a nickname to a set of commands.{}
: These curly braces tell Bash that the following lines belong to your function.# commands
: This is where you put the commands you want to include in your function.
Example: A Friendly Greeting
Let's create a simple function that prints a friendly greeting message. Open your terminal and type this:
greet() {
echo "Hello, $1!"
}
greet
: This is the name of our function.$1
: This is a special variable that represents the first argument you give to your function.
Now, let's use our new function:
greet Alice
Output:
Hello, Alice!
See how easy it is to use our function? We just typed greet Alice
and it printed our greeting message!
Adding Extra Power: Conditional Statements and Loops
Your functions can do even more amazing things by using conditional statements and loops.
Example: A Helpful Backup Function
Creating backups is important to keep your data safe. Let's build a function to help us with that:
backup_file() {
if [ -f "$1" ]; then
cp "$1" "$1.bak"
echo "Backup of $1 created as $1.bak."
else
echo "File $1 does not exist."
fi
}
Let's break down the code:
if [ -f "$1" ]; then ... fi
: This part checks if the file given as the first argument ($1
) exists.cp "$1" "$1.bak"
: If the file exists, this command copies the file to a new file with the name$1.bak
.echo "Backup of $1 created as $1.bak."
: This line prints a success message telling us that the backup was created.else ... fi
: If the file doesn't exist, this part prints an error message.
Now, let's use our backup function:
backup_file my_important_document.txt
If my_important_document.txt
exists, a backup called my_important_document.txt.bak
will be created, and you'll see a success message. If not, you'll see an error message.
Example: Counting Lines in a File
Let's create a function to count how many lines are in a text file:
count_lines() {
if [ -f "$1" ]; then
local COUNT=$(wc -l < "$1")
echo "The file '$1' has $COUNT lines."
else
echo "File $1 does not exist."
fi
}
Here's what's happening:
local COUNT=$(wc -l < "$1")
: This line stores the number of lines in the file ($1
) into a variable calledCOUNT
.echo "The file '$1' has $COUNT lines."
: This line prints the count of lines.
Let's try it out:
count_lines my_file.txt
If my_file.txt
exists, you'll see a message like "The file 'my_file.txt' has 20 lines."
Handling Multiple Arguments and Complex Scenarios
What if we need our functions to work with multiple inputs? Let's explore how to handle more complex scenarios.
Example: Copy and Rename a File
Imagine you want to copy a file and give it a new name. Here's a function to do that:
copy_and_rename() {
if [ -f "$1" ]; then
cp "$1" "$2"
echo "File $1 copied to $2."
else
echo "File $1 does not exist."
fi
}
Here's how it works:
$1
: Represents the original file name.$2
: Represents the new file name.
To use this function:
copy_and_rename old_file.txt new_file.txt
Output:
File old_file.txt copied to new_file.txt.
Example: Cleaning Up Temporary Files
Let's create a function to remove all temporary files (.tmp
files) from a directory:
cleanup_tmp_files() {
local DIR="$1"
if [ -d "$DIR" ]; then
rm -f "$DIR"/*.tmp
echo "Temporary files in $DIR have been cleaned up."
else
echo "Directory $DIR does not exist."
fi
}
local DIR="$1"
: This line stores the directory name given as the argument ($1
) into a variable calledDIR
.rm -f "$DIR"/*.tmp
: This command removes all files ending with.tmp
in the specified directory.
To use this function:
cleanup_tmp_files /path/to/my/directory
Output:
Temporary files in /path/to/my/directory have been cleaned up.
Tips for Creating Awesome Bash Functions
Here are some helpful tips to make your Bash functions even better:
- Use
local
Variables: When you create variables inside a function, use thelocal
keyword. This helps to prevent any confusion with variables that might have the same name outside the function. - Handle Errors Gracefully: Things don't always go as planned! Add checks and error messages to your function to handle unexpected situations.
- Write Helpful Comments: Add comments to your code to explain what your function does. This will make your code easier to understand, both for you and for anyone else who might need to use it.
Example: A Function with Error Handling and Comments
Let's create a function that compresses a file and checks if the compression worked:
compress_file() {
# Check if the file exists
if [ ! -f "$1" ]; then
echo "File $1 does not exist."
return 1
fi
# Compress the file using tar
tar -czf "$1.tar.gz" "$1"
# Check if the compression was successful
if [ $? -eq 0 ]; then
echo "File $1 successfully compressed."
else
echo "Compression failed for $1."
fi
}
if [ ! -f "$1" ]; then ... fi
: Checks if the file exists. If not, it prints an error message and exits the function.return 1
: This tells Bash that the function encountered an error.tar -czf "$1.tar.gz" "$1"
: This command compresses the file into a.tar.gz
archive.if [ $? -eq 0 ]; then ... fi
: Checks if the compression command was successful. The$?
variable holds the exit status of the last command.
Now, let's try it:
compress_file my_document.txt
You'll either see a success message or an error message based on whether the compression worked or not.
Conclusion: Bash Functions Are Your Power Tools
Creating custom Bash functions can make your command line experience much smoother and more efficient. By automating repetitive tasks, organizing complex commands, and writing clear and reusable code, Bash functions can help you work smarter, not harder.
Start by defining simple functions and gradually add complexity as you gain confidence. The key is to identify tasks that you perform frequently and write functions to automate them.
You've got this! Happy scripting!