Efficient File Manipulation with Bash: A Beginner's Guide
Master file manipulation with Bash scripting! This beginner's guide covers essential commands, from creating & deleting files to batch renaming and advanced text processing. Learn to automate repetitive tasks, save time, and streamline your workflow.
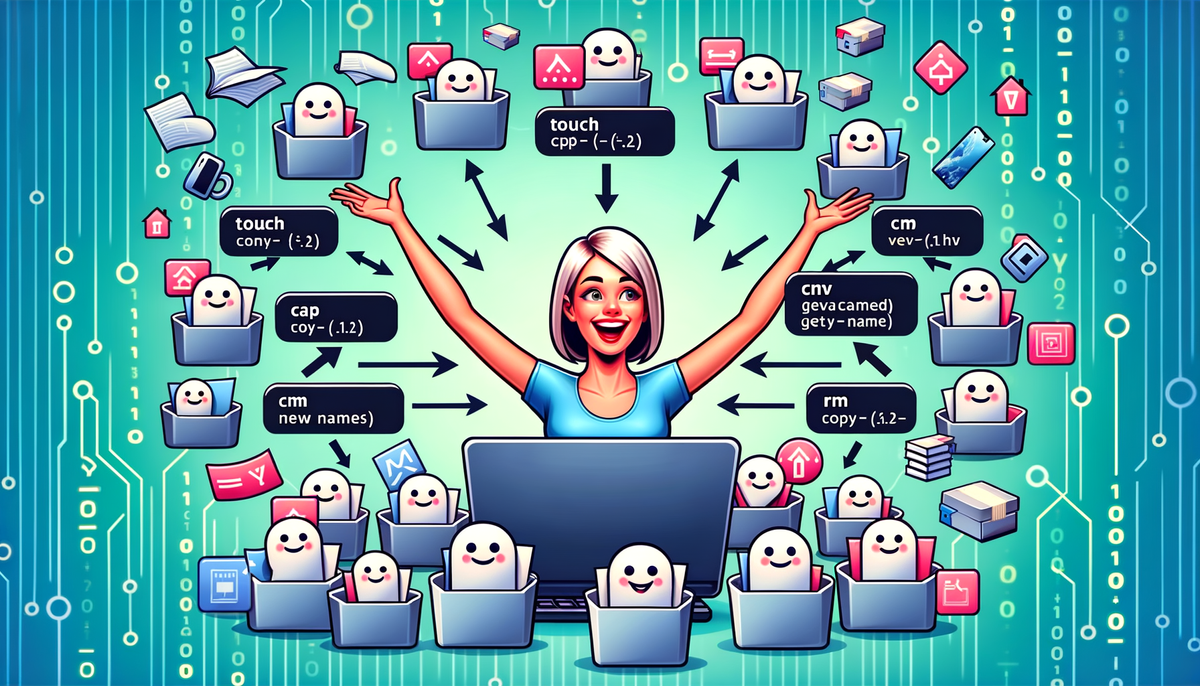
Are you tired of manually managing hundreds of files on your Linux system? Do you find yourself repeating the same file operations again and again? If so, Bash scripting can be your best friend.
This guide will teach you how to use Bash commands to efficiently manipulate files. We'll cover everything from basic operations like creating and deleting files to more advanced techniques like batch renaming and filtering. By the end, you'll be able to automate tedious tasks and save yourself countless hours of manual work.
Why Use Bash for File Manipulation?
Bash is a powerful shell that's widely used in Linux and other Unix-like systems. Here's why it's a great choice for file manipulation:
- Efficiency: Bash allows you to perform complex operations on multiple files with a single command, saving you a lot of time.
- Automation: You can create scripts to automate repetitive tasks, freeing you up to focus on other things.
- Flexibility: Bash provides a wide range of commands and options, making it possible to handle almost any file manipulation task.
Basic Bash Commands for File Manipulation
Let's start with some basic commands that form the foundation of more complex scripts.
1. Creating Files
The touch
command creates a new, empty file. For example:
touch myfile.txt
This creates a file named myfile.txt
. If the file already exists, touch
will update its timestamp.
2. Viewing File Contents
The cat
command displays the contents of a file on the terminal. For example:
cat myfile.txt
This will show the contents of the myfile.txt
file. For large files, it's better to use less
or more
to scroll through the output.
3. Copying and Moving Files
The cp
command copies files, while the mv
command moves (or renames) files. Here's how they work:
# Copy myfile.txt to newfile.txt
cp myfile.txt newfile.txt
# Move myfile.txt to another directory or rename it
mv myfile.txt documents/myfile.txt
The cp
command creates a duplicate of the source file. The mv
command moves the file to a different location or changes its name.
4. Deleting Files
The rm
command removes files. Use it with caution, as it permanently deletes files:
rm myfile.txt
This will delete the myfile.txt
file. If you want to delete multiple files, use wildcards. For example, rm *.txt
will delete all files with the .txt
extension in the current directory.
Advanced File Manipulation with Bash
Now let's dive into more advanced techniques that can save you even more time.
1. Batch Renaming Files
Imagine you have a bunch of images named img1.jpg
, img2.jpg
, and so on. You want to rename them to photo1.jpg
, photo2.jpg
, etc. This can be easily done with a Bash script:
for file in img*.jpg; do
newname="photo${file#img}"
mv "$file" "$newname"
done
This script loops through each file starting with "img" and ending with ".jpg". It then constructs a new name by replacing "img" with "photo". Finally, it renames the file using the mv
command.
2. Finding and Deleting Files
The find
command is essential for locating specific files. You can use it to find files based on their name, type, modification date, and other criteria.
For example, to delete all .tmp
files in the current directory and its subdirectories:
find . -type f -name "*.tmp" -delete
This command searches for files with the .tmp
extension (using -name
) and deletes them (using -delete
).
3. Processing Text Files
Let's say you have a file called names.txt
containing a list of names, and you want to create a separate file for each name. Here's how you can do it:
while IFS= read -r name; do
touch "${name}.txt"
done < names.txt
This script reads each line from names.txt
and uses the touch
command to create a file with the name on that line.
Automating Tasks with Cron Jobs
Sometimes you want to run Bash scripts automatically at specific times. This is where cron jobs come in.
To create a cron job, open the cron table using crontab -e
. Then, add a line like this:
0 0 * * * /path/to/your/script.sh
This will run your/script.sh
every day at midnight (00:00). You can customize the schedule to run the script at different times or intervals.
Advanced Bash Techniques
Let's explore some more advanced techniques that can come in handy for specific tasks.
1. Merging Files
The cat
command can be used to merge multiple files into one. For example:
cat file1.txt file2.txt > merged_file.txt
This will combine the contents of file1.txt
and file2.txt
into a new file called merged_file.txt
.
2. Sorting File Contents
The sort
command sorts the lines of a file alphabetically. For example:
sort names.txt -o sorted_names.txt
This will sort the lines in names.txt
and save the result to sorted_names.txt
.
3. Filtering File Contents
The grep
command searches for lines containing a specific pattern. For example:
grep "Alice" names.txt
This will display all lines in names.txt
that contain the word "Alice".
Practical Examples
Let's put everything together with a couple of practical examples.
1. Backup Script
This script backs up all .txt
files in the current directory to a directory named after the current date:
#!/bin/bash
backup_dir="backup_$(date +%Y%m%d)"
mkdir "$backup_dir"
cp *.txt "$backup_dir"
echo "Backup completed in $backup_dir"
Save this script as backup.sh
and make it executable with chmod +x backup.sh
. Then, run it to create a backup of your .txt
files.
2. Log File Cleanup
This script compresses and moves old log files to a backup directory:
#!/bin/bash
find /var/log -type f -name "*.log" -mtime +30 -exec gzip {} \;
find /var/log -type f -name "*.log.gz" -exec mv {} /backup/logs \;
echo "Log files cleaned up and moved to /backup/logs"
This script uses find
to locate log files older than 30 days, compresses them with gzip
, and moves the compressed files to a backup directory.
Conclusion
Bash is a powerful tool for efficient file manipulation. By learning these basic and advanced techniques, you can automate tasks, save time, and keep your file system organized. Don't be afraid to experiment and practice. The more you use Bash, the more comfortable and efficient you'll become.
Happy scripting!