GoLang for Beginners: A Comprehensive Guide to Getting Started
Learn GoLang, Google's fast and efficient programming language, from scratch. This beginner-friendly guide covers installation, basic syntax, control structures, modules, functions, structs, and error handling. Start building reliable software today!
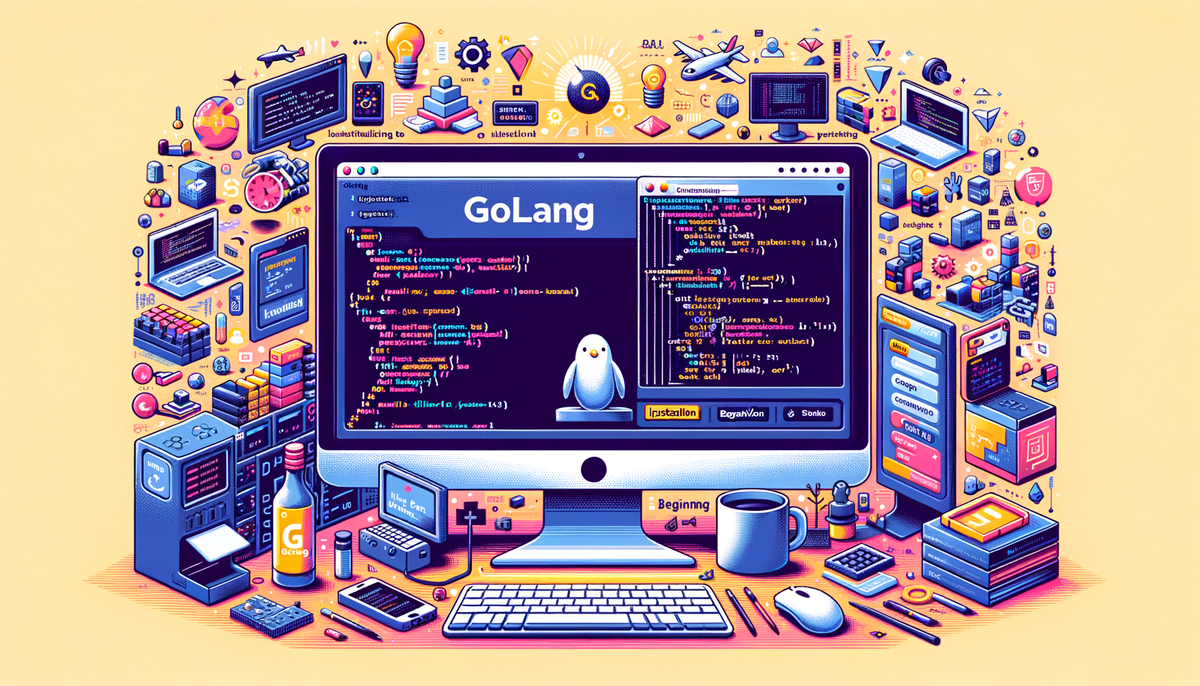
Welcome to our comprehensive guide on GoLang, a simple and efficient programming language created by Google. Whether you're new to programming or looking to add another language to your tool belt, this guide will help you kickstart your GoLang journey with ease.
Why Learn GoLang?
GoLang, also known as Go, was developed by Google to make it easy to build reliable and efficient software. Major tech companies like Netflix and Dropbox use it because of its simple syntax and fast performance. GoLang is perfect for web development, cloud computing, and server-side projects. Its straightforward design makes it a great choice for beginners, as you can quickly solve complex problems using Go.
Getting Started with GoLang
Installing GoLang
Before we start coding, you need GoLang on your computer. Here are the steps:
-
Download GoLang: Visit the official GoLang Download Page and choose the version for your operating system (Windows, macOS, or Linux).
-
Install GoLang: Download the installer and follow the instructions. For Windows, run the
.msi
file. macOS users can open the.pkg
file. Linux users can follow the Terminal commands provided on the download page. -
Verify Installation: Open your terminal or command prompt and type:
go version
If GoLang is installed correctly, you'll see the Go version you installed.
Setting Up Your First GoLang Program
Now that GoLang is installed, let's write our first program:
-
Create a Project Directory: Create a new folder for your Go project. In your terminal, navigate to your desired location and create a folder:
mkdir hello_go cd hello_go
-
Create a Go File: Inside your project directory, create a file named
main.go
using your preferred text editor (Notepad, VS Code, etc.). -
Write Your First Program: Open
main.go
and enter the following code:package main import "fmt" func main() { fmt.Println("Hello, World!") }
Running Your Go Program
To run your GoLang program, navigate to your project directory in the terminal and type:
go run main.go
You should see the output:
Hello, World!
Congratulations! You've written and run your first Go program!
Understanding the Basics
Go Modules
Go modules are collections of related Go packages. They help manage dependencies in your projects. Think of it like having organized boxes for your building blocks!
-
Initialize a Go Module: Use the
go mod
command to create a new module:go mod init hello_go
This creates a
go.mod
file in your project directory, which tracks the dependencies of your project. -
Creating Packages: Packages are groups of related functions. To create one, simply create a new file within your project directory:
// Create a file called greet.go package greet import "fmt" func Hello() { fmt.Println("Hello from the greet package!") }
-
Using Packages: To use your new
greet
package in yourmain.go
file, you need to import it:package main import ( "fmt" "hello_go/greet" // Import the greet package ) func main() { fmt.Println("Starting main program") greet.Hello() }
This allows you to use the
Hello
function from thegreet
package in yourmain
function.
Control Structures
GoLang provides familiar control structures like if
, for
, and switch
for controlling the flow of your program.
If Else Statement
package main
import "fmt"
func main() {
x := 10
if x > 5 {
fmt.Println("x is greater than 5")
} else {
fmt.Println("x is less than or equal to 5")
}
}
This code checks if the variable x
is greater than 5. If it is, it prints "x is greater than 5." Otherwise, it prints "x is less than or equal to 5."
For Loop
package main
import "fmt"
func main() {
for i := 0; i < 5; i++ {
fmt.Println(i)
}
}
This code uses a for
loop to print the numbers 0 through 4. It initializes the variable i
to 0, then continues to print i
and increment it by 1 until it reaches 5.
Switch Case
package main
import "fmt"
func main() {
day := "Monday"
switch day {
case "Monday":
fmt.Println("Start of the work week")
case "Saturday", "Sunday":
fmt.Println("It's the weekend!")
default:
fmt.Println("Midweek days")
}
}
This code uses a switch
statement to check the value of the day
variable. It checks if day
is equal to "Monday", "Saturday", or "Sunday." If it matches any of these cases, it prints the corresponding message. If it doesn't match any of these cases, it prints "Midweek days."
Key GoLang Concepts
Functions
Functions are reusable blocks of code that perform specific tasks. Think of them like tools in a toolbox – each tool has a specific purpose.
package main
import "fmt"
func add(x int, y int) int {
return x + y
}
func main() {
result := add(3, 5)
fmt.Println(result) // Output: 8
}
This code defines a function called add
that takes two integers as input and returns their sum. The main
function then calls the add
function with the values 3 and 5 and prints the result.
Structs
Structs are custom data types that can hold different pieces of information. Imagine a box that can hold specific items, like a name, age, or address.
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
person := Person{Name: "Alice", Age: 25}
fmt.Println(person)
}
This code defines a Person
struct with two fields: Name
and Age
. The main
function then creates a Person
variable called person
and initializes its fields. It then prints the person
variable, which will output the name and age.
Error Handling
Error handling in Go is explicit and simple. It helps you catch and manage errors that might occur during your program's execution.
package main
import (
"errors"
"fmt"
)
func divide(a, b float64) (float64, error) {
if b == 0 {
return 0, errors.New("division by zero")
}
return a / b, nil
}
func main() {
result, err := divide(5, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
This code defines a divide
function that divides two numbers. It checks if the denominator (b
) is 0, and if so, returns an error. The main
function then calls the divide
function and checks for errors. If there's an error, it prints the error message; otherwise, it prints the result.
Conclusion
You've learned the basics of GoLang, including how to install it, write simple programs, and understand key concepts. GoLang's simplicity and efficiency make it a powerful tool for various applications. Start experimenting with more complex projects to fully harness the power of GoLang. Happy coding!
Remember, practice makes perfect. The more you code, the better you'll become. Stay curious and keep learning!