GoLang Performance Optimization: Tips and Tricks
Boost your GoLang application's speed with these essential optimization techniques. Learn how to profile code, manage memory efficiently, use concurrency wisely, and employ best practices for data structures, strings, and JSON handling. Unlock the full potential of your Go code today!
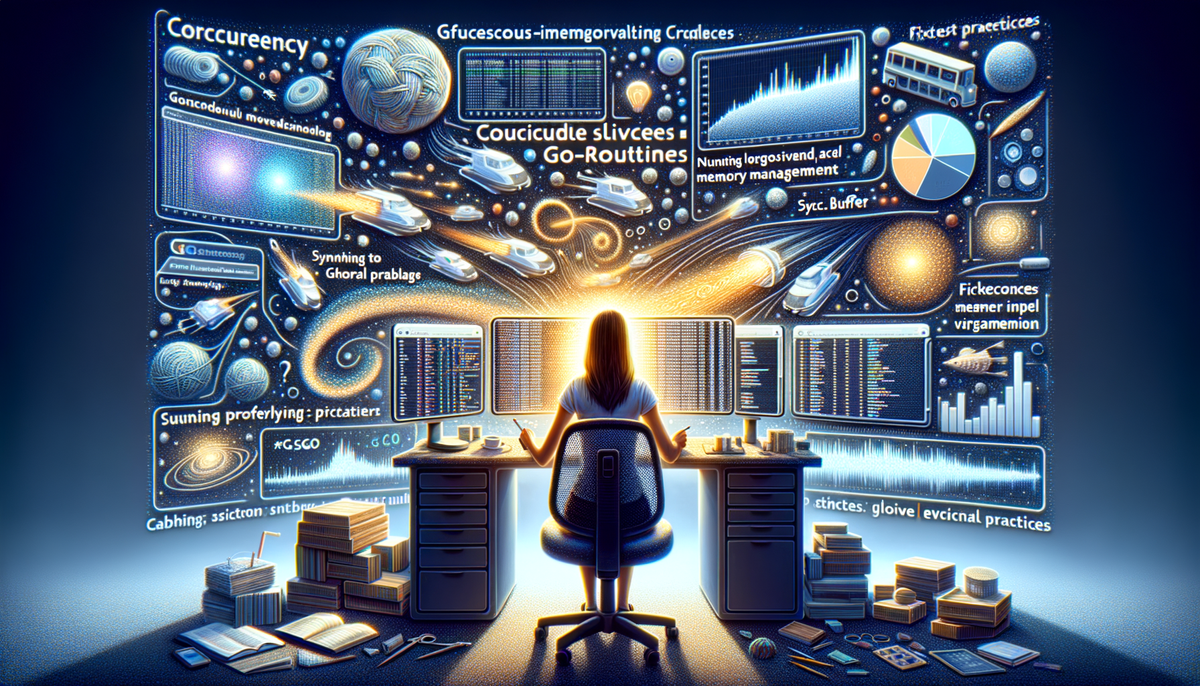
GoLang, also known as Go, is a popular programming language known for its speed and simplicity. However, to fully leverage its potential, it is essential to ensure that your Go code runs as efficiently as possible. This guide provides tips and tricks to optimize the performance of your Go programs by covering important aspects such as memory usage, concurrency, and coding best practices.
Why Optimize GoLang?
Optimizing your Go code can solve various issues encountered during development. Slow code can lead to unhappy users and resource wastage on your server. Enhancing the performance of your code ensures the smooth functioning of your program and increases user satisfaction.
Use Profiling Tools
Profiling tools are essential for identifying performance bottlenecks in your code. One of the most effective tools in Go is pprof
.
Example:
-
Import the necessary packages:
import ( "net/http" _ "net/http/pprof" )
-
Start the pprof server for profiling:
go func() { log.Println(http.ListenAndServe("localhost:6060", nil)) }()
-
Run your program and navigate to
http://localhost:6060/debug/pprof
in your web browser to view the profiling data.
Optimize Memory Usage
Efficient memory usage is crucial for performance. Carefully manage slices, as they can consume excessive memory and degrade performance.
Example:
- Preallocate slice capacity when possible:
// Inefficient: var data []int for i := 0; i < 1000; i++ { data = append(data, i) } // Efficient: data := make([]int, 0, 1000) for i := 0; i < 1000; i++ { data = append(data, i) }
Use Concurrency Wisely
Go excels in handling concurrent tasks, but improper use can lead to performance issues. Ensure proper synchronization and avoid race conditions.
Example:
- Using goroutines:
func process(data int) { fmt.Println(data) } func main() { for i := 0; i < 100; i++ { go process(i) } time.Sleep(time.Second) // Wait for all goroutines to finish }
Avoid Global Variables
Global variables can complicate code maintenance and introduce bugs, particularly in concurrent programs. Prefer local variables inside functions.
Example:
-
Avoid global variables:
var result int func add(a, b int) { result = a + b }
-
Use local variables:
func add(a, b int) int { return a + b }
Minimize Allocations
Frequent memory allocations can slow down your program. Reuse memory whenever possible.
Example:
- Use
sync.Pool
for reusable objects:import "sync" type MyStruct struct{} var pool = sync.Pool{ New: func() interface{} { return new(MyStruct) }, } obj := pool.Get().(*MyStruct) // use obj pool.Put(obj)
Efficient String Operations
String manipulation can be slow. Opt for methods that avoid unnecessary allocations, like using bytes.Buffer
.
Example:
-
Inefficient concatenation:
s := "Hello" + " " + "World"
-
Efficient using
bytes.Buffer
:import "bytes" var buffer bytes.Buffer buffer.WriteString("Hello") buffer.WriteString(" ") buffer.WriteString("World") s := buffer.String()
Use Proper Data Structures
Choosing the right data structure can significantly enhance performance. Use arrays, slices, maps, and structs appropriately based on your requirements.
Example:
-
Map usage:
var data = map[int]int{1: 1, 2: 2, 3: 3}
-
Slice usage:
data := []int{1, 2, 3}
Cache for Repeated Computations
Caching can save time by reusing previously computed results.
Example:
- Implementing a cache for Fibonacci numbers:
var cache = make(map[int]int) func fibonacci(n int) int { if n <= 1 { return n } if val, found := cache[n]; found { return val } cache[n] = fibonacci(n-1) + fibonacci(n-2) return cache[n] } result := fibonacci(10) // Fast subsequent retrievals
Avoid Memory Leaks
Prevent memory leaks by properly managing resources, especially when using goroutines, channels, and servers.
Example:
- Properly closing channels:
func main() { jobs := make(chan int, 5) finished := make(chan bool) go func() { for { job, more := <-jobs if more { fmt.Println("Received job", job) } else { fmt.Println("All jobs received, terminating goroutine") finished <- true return } } }() for j := 1; j <= 3; j++ { jobs <- j fmt.Println("Sent job", j) } close(jobs) fmt.Println("Sent all jobs") <-finished }
Use Efficient JSON Handling
JSON parsing can be a performance bottleneck. Use json.Decoder
for large data sets to avoid creating large structs.
Example:
- Efficient JSON decoding:
import ( "encoding/json" "os" ) type MyStruct struct{} func main() { file, _ := os.Open("data.json") defer file.Close() decoder := json.NewDecoder(file) for decoder.More() { var data MyStruct decoder.Decode(&data) } }
Conclusion
Optimizing GoLang code can significantly improve program performance, leading to happier users and resource savings. Use profiling tools to identify slow code, manage memory effectively, utilize concurrency properly, avoid global variables, minimize allocations, optimize string operations, choose the right data structures, cache expensive computations, and handle JSON data efficiently. By adopting these tips and tricks, you can ensure your Go programs run at their best.
Happy coding!