GoLang vs. Python: Which Language Should You Learn?
Choosing between GoLang and Python? GoLang excels at speed and efficiency, ideal for building scalable applications. Python's ease of learning makes it perfect for data science, machine learning, and web development. This article helps you decide which language aligns with your goals.
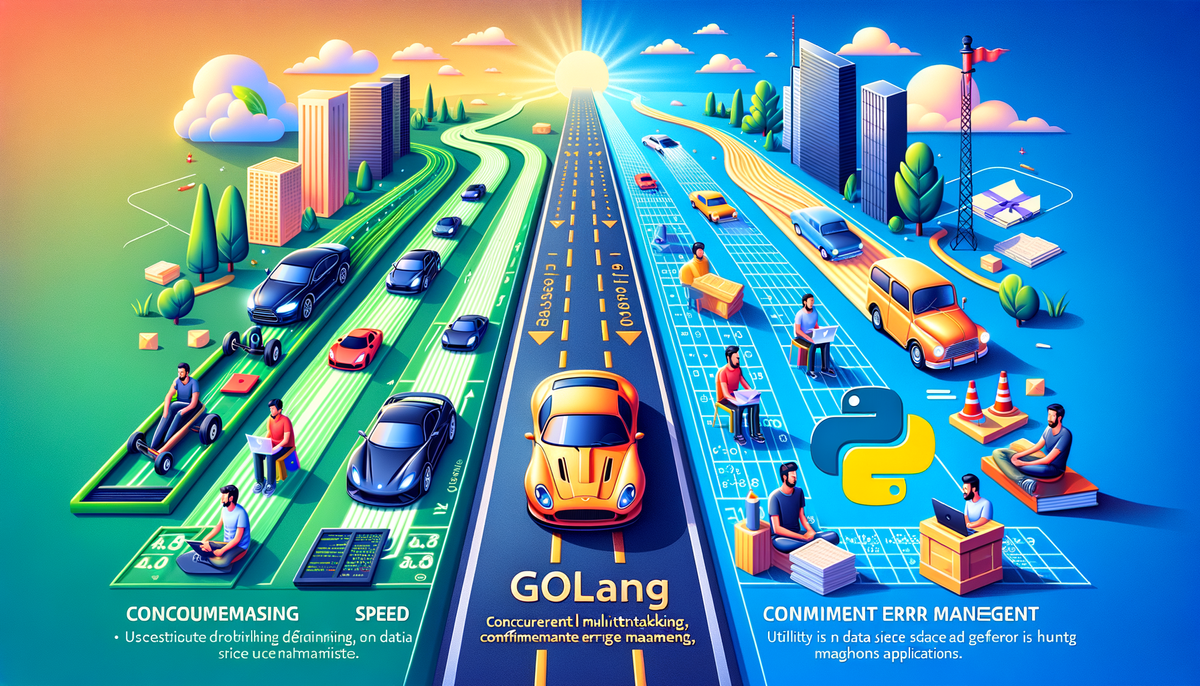
Choosing the right programming language can be tough. There are many options available, each with its own strengths and weaknesses. Two popular choices are GoLang (also known as Go) and Python. This article will help you decide which language is right for you.
GoLang: Built for Speed and Efficiency
GoLang is a language created by Google that is known for being fast and good at handling many tasks at once. It's like a race car that can handle lots of traffic.
Here's a simple example of how you can print "Hello, World!" in GoLang:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
This code creates a program that will print "Hello, World!" to the screen.
Python: Easy to Learn and Use
Python is known for being easy to learn, even for beginners. It's like a friendly guide that helps you learn to code without getting lost.
Here's a simple example of how you can print "Hello, World!" in Python:
print("Hello, World!")
This code does the same thing as the GoLang code, but it's even shorter.
Performance: Which Language is Faster?
GoLang is usually faster than Python. This is because GoLang is like a compiled language, which means it turns your code into instructions the computer can understand directly. Python is like an interpreted language, which means it reads your code line by line and then executes it.
Here's a simple example of how to add two numbers in both languages:
GoLang: Adding Numbers
package main
import "fmt"
func add(a int, b int) int {
return a + b
}
func main() {
fmt.Println(add(3, 5)) // Output: 8
}
Python: Adding Numbers
def add(a, b):
return a + b
# Example usage
print(add(3, 5)) # Output: 8
Both languages do the same thing, but GoLang is usually faster.
Learning Curve: Which Language is Easier to Learn?
Python is generally considered easier to learn for beginners. Its syntax is simple and easy to understand, just like speaking English.
Use Cases: Where Each Language Shines
GoLang is a great choice for:
- Building fast websites and apps
- Working with large amounts of data
- Creating software for cloud computing
Python is a great choice for:
- Data science (analyzing and understanding data)
- Machine learning (teaching computers to learn)
- Web development (creating websites)
Community and Libraries
Python has a larger community of developers, which means there are many resources and libraries available to help you. GoLang has a growing community and many helpful libraries as well.
Error Handling
GoLang requires you to handle errors explicitly, which makes your code more reliable but also more verbose.
GoLang: Error Handling
package main
import (
"errors"
"fmt"
)
func divide(a, b float64) (float64, error) {
if b == 0 {
return 0, errors.New("divide by zero")
}
return a / b, nil
}
func main() {
result, err := divide(4, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
In Python, exceptions make the code cleaner but can be harder to manage.
Python: Error Handling
def divide(a, b):
try:
return a / b
except ZeroDivisionError:
return "divide by zero error"
# Example usage
print(divide(4, 0)) # Output: divide by zero error
Conclusion: Which Language is Right for You?
Choosing between GoLang and Python depends on your goals:
- If you need a fast and efficient language for building scalable applications, GoLang is a great choice.
- If you need a simple and easy-to-learn language for data science, machine learning, or web development, Python is a great choice.
No matter which language you choose, you'll have a strong community and helpful resources to support you. Good luck with your coding journey!