How Golang Manages Memory: A Deep Dive into Stack and Heap
Dive into Golang's stack and heap memory management to enhance application performance and efficiency! This guide explores Golang's unique approach, automatic garbage collection, and offers practical memory optimization tips. Master your Golang memory game for peak performance.
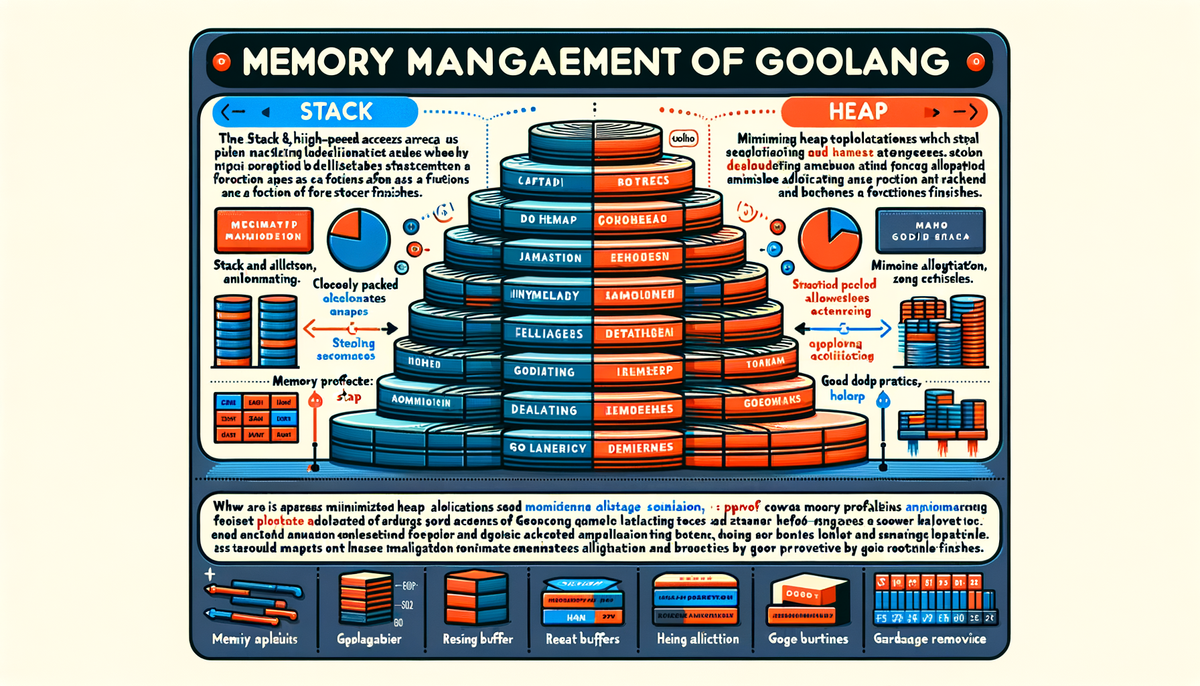
Memory management is a key component of writing efficient code in any programming language. Golang, known for its simplicity and performance, has a unique way of handling memory which is crucial for ensuring your programs run smoothly without unnecessary resource consumption. This guide will provide an in-depth look at how Golang uses stack and heap memory, enhancing your understanding and expertise in memory management.
Why Memory Management Matters
Understanding how memory is managed helps in writing fast, stable, and efficient applications. Efficient memory use prevents crashes and improves performance. By grasping the stack and heap in Golang, you can optimize your application's memory usage.
Stack vs. Heap: The Basics
Stack Memory
- Definition: The stack is where temporary variables are stored. When a function is called, a block of memory is reserved for its local variables and control data.
- Characteristics:
- Size Limit: Limited size, which ensures speed and efficiency for small variables.
- Access Speed: Fast access due to CPU management.
- Scope: Variables get automatically deallocated once the function finishes.
Heap Memory
- Definition: The heap is used for dynamic memory allocation. Unlike the stack, memory on the heap persists until it is manually deallocated.
- Characteristics:
- Size Flexibility: Larger and more flexible than the stack.
- Access Speed: Slower access as it utilizes pointers.
- Manual Management: Requires explicit allocation and deallocation, though Golang's garbage collector assists with this process.
Golang's Approach to Memory Management
Golang blends automatic and manual memory management for simplicity and safety.
Stack Allocation
Simple variables such as integers and floats typically reside in the stack. Here's an example:
func stackExample() {
var x int = 10 // 'x' is stored on the stack
}
Here, x
exists only during the execution of stackExample
and is automatically cleaned up when the function ends.
Heap Allocation
More complex structures or larger data may require heap allocation. Golang decides based on the context when heap allocation is needed:
func heapExample() *int {
x := new(int) // 'x' is allocated on the heap
*x = 10
return x
}
In heapExample
, the integer at which x
points remains allocated after the function exits, allowing program-wide use.
Golang's Garbage Collection
Golang's garbage collector helps manage heap-allocated memory by automatically freeing up memory space when objects go out of use, minimizing memory leaks and reducing programmer workload.
Benefits of Garbage Collection
- Ease of Use: Eases the burden of manual memory management.
- Safety: Prevents memory leaks which can destabilize applications.
Profiling Memory in Golang
Profiling helps visualize memory usage, aiding optimization. Golang provides tools like pprof
to help you profile and analyze memory use effectively.
Using pprof
pprof
enables analysis of memory consumption. Here's how to leverage it:
- Import
runtime/pprof
: Include the profiling package. - Start Profiling: Use
pprof
to record memory usage data. - Analyze Output: Inspect the results to identify memory usage patterns and inefficiencies.
package main
import (
"log"
"runtime/pprof"
"os"
)
func main() {
f, err := os.Create("memprofile")
if err != nil {
log.Fatal(err)
}
defer f.Close()
pprof.WriteHeapProfile(f)
}
This snippet writes a memory profile to a file for detailed analysis.
Practical Tips for Memory Optimization
- Minimize Heap Allocations: Prefer stack memory where possible to lessen garbage collection needs.
- Profile Often: Regularly use tools like
pprof
to spot and correct inefficiencies. - Manage Goroutines: Avoid goroutines that cling to memory unnecessarily.
- Reuse Buffers: Reuse memory spaces to cut down on allocations.
Conclusion
Mastering Golang's memory management is pivotal for writing high-performance applications. By understanding stack and heap allocations and employing tools like garbage collection and pprof
, you can optimize memory usage significantly. With these insights, you're equipped to create reliable and efficient Golang applications. Happy coding!