How to Handle Multithreading in Go: A Step-by-Step Guide
Unlock the power of Go's concurrency with our step-by-step guide! Learn to leverage goroutines, channels, and WaitGroups for efficient multithreading. Master error handling and synchronization to create responsive, robust applications. Dive into Go's concurrent capabilities today!
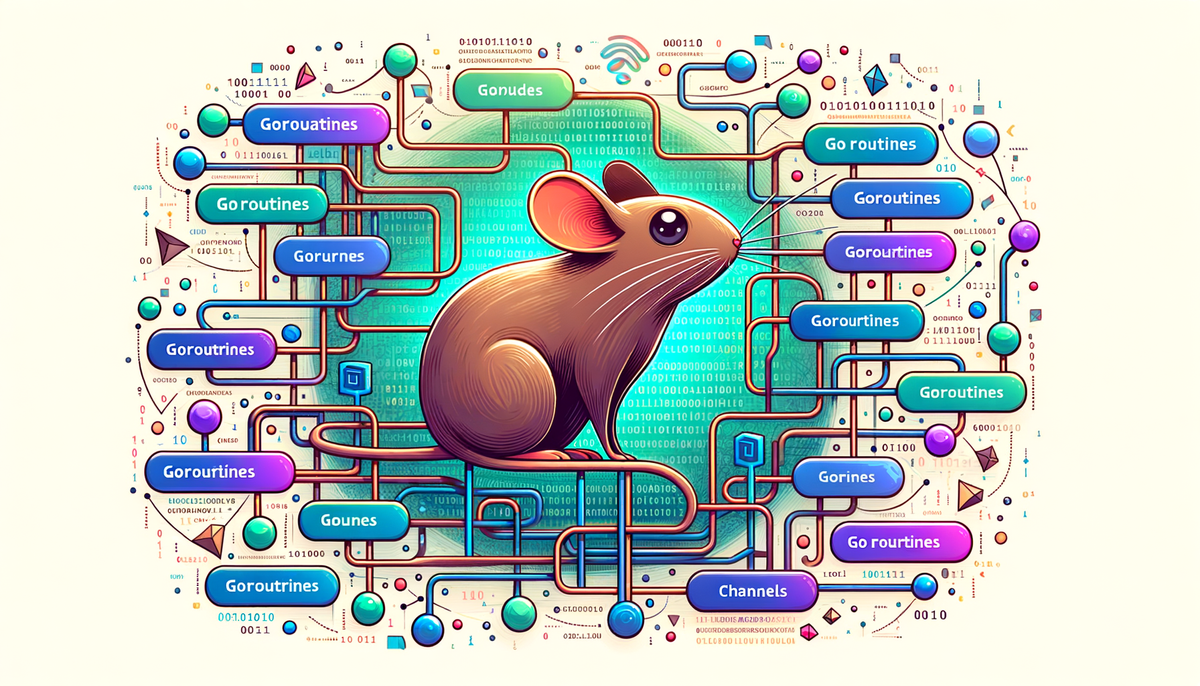
Understanding how to manage concurrency in your applications is crucial for building efficient, responsive software. Go, also known as Golang, is a language designed with concurrency in mind, offering several features that make multithreading easier and more effective. This guide, equipped with examples and step-by-step tutorials, will help you learn "how to handle multithreading in Go."
Introduction
Concurrency allows multiple tasks to execute at the same time, leading to better performance and resource utilization. In this "Go routine concurrency tutorial," we'll dive into the basics of handling multithreading in Go, provide practical examples with channels and goroutines, and help you gain a solid understanding of Go's concurrent capabilities.
Why Concurrency Matters
Concurrency is increasingly important in modern software development. By enabling tasks to run simultaneously, applications can perform a variety of functions without lagging or becoming unresponsive. Go offers goroutines and channels as easy-to-use tools for implementing concurrency.
Understanding Goroutines
What is a Goroutine?
Goroutines are essentially threads managed by the Go runtime. They're cheap to run, easy to handle, and core to Go's concurrency model. Goroutines allow you to start new tasks and have them run alongside other tasks seamlessly.
Example: Launching a Simple Goroutine
Here's how you can start a simple goroutine in Go:
package main
import (
"fmt"
"time"
)
func sayHello() {
fmt.Println("Hello, world!")
}
func main() {
go sayHello() // launch a goroutine
time.Sleep(time.Second)
fmt.Println("Goodbye!")
}
How Goroutines Work
- Function Prefix: To start a function as a goroutine, simply prefix it with the
go
keyword. - Concurrency: Goroutines allow functions to execute concurrently, leading to better resource utilization.
Using Channels for Communication
What are Channels?
Channels in Go act like pipes—goroutines send and receive data through these channels. They are both typed and thread-safe, ensuring reliable communication.
Example: Basic Channel Usage
Here's an example of how to use basic channels in Go:
package main
import (
"fmt"
)
func sendMessage(ch chan string) {
ch <- "Hello, Go!"
}
func main() {
ch := make(chan string)
go sendMessage(ch)
msg := <-ch
fmt.Println(msg)
}
How Channels Work
- Channel Creation: Use
make(chan type)
to create a channel, specifying the type for data transfer. - Sending and Receiving: Use
<-
to send (ch <- data
) and receive (data := <-ch
) data through the channel.
Synchronizing Goroutines
Using WaitGroup
The sync.WaitGroup
type in Go is used to wait for a collection of goroutines to finish executing. It simplifies managing concurrent operations.
Example: Using WaitGroup
package main
import (
"fmt"
"sync"
)
func printNumber(num int, wg *sync.WaitGroup) {
fmt.Println(num)
wg.Done()
}
func main() {
var wg sync.WaitGroup
for i := 1; i <= 5; i++ {
wg.Add(1)
go printNumber(i, &wg)
}
wg.Wait()
fmt.Println("All goroutines finished.")
}
Efficient Synchronization
- Add: Call
wg.Add(n)
to addn
to the WaitGroup's counter. - Done: Call
wg.Done()
once for each goroutine to decrease the counter. - Wait: Use
wg.Wait()
to block until the counter is zero.
Error Handling in Goroutines
When using goroutines, traditional error handling can become complex. Channels offer a way to propagate errors back to the main routine efficiently.
Example: Error Handling with Channels
package main
import (
"errors"
"fmt"
)
func riskyTask(ch chan error) {
performTask := false
if !performTask {
ch <- errors.New("Task failed")
return
}
ch <- nil
}
func main() {
errorChan := make(chan error)
go riskyTask(errorChan)
if err := <-errorChan; err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Task succeeded")
}
}
Key Takeaways
- Error Channels: Incorporate a dedicated error channel to handle issues gracefully between goroutines and the main function.
Conclusion
You've now explored a comprehensive "Go thread handling example" using goroutines, channels, and synchronization tools. These features allow you to take full advantage of Go's concurrency model and build powerful, efficient applications. By further experimenting and applying these concepts, you'll consistently improve your handling of multithreading challenges in Go.