How to Run a Bash Script as a Command: A Comprehensive Guide
Unlock the power of bash scripting! Learn how to create, run, and turn scripts into commands. From basics to advanced techniques, this guide covers variables, loops, functions, and more. Boost your productivity and automate tasks with ease. Start your scripting journey today!
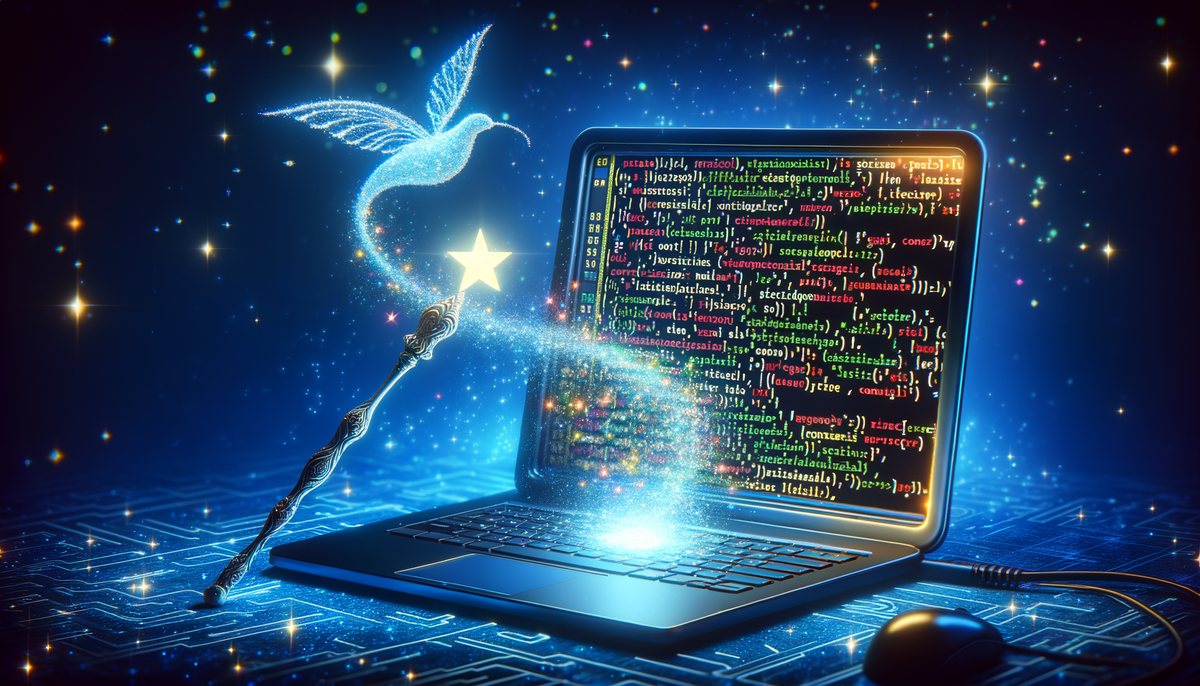
Are you tired of typing long commands in your terminal? Want to automate repetitive tasks? Learning how to run a bash script as a command is your solution! This guide will walk you through the process, from creating your first script to running it like any other command on your system. We'll cover everything you need to know about bash scripting, from the basics to more advanced concepts.
What is a Bash Script?
A bash script is a text file containing a series of commands that the bash shell can execute. It's like a recipe for your computer, telling it exactly what to do step by step. Bash scripts are powerful tools for automating tasks, managing systems, and streamlining your workflow.
Creating Your First Bash Script
Let's start with a simple example:
- Open your favorite text editor
- Type the following:
#!/bin/bash
echo "Hello, World!"
- Save the file as
hello.sh
The first line #!/bin/bash
is called a shebang. It tells the system to use bash to interpret the script.
Making Your Script Executable
Before you can run your script, you need to make it executable:
- Open your terminal
- Navigate to the directory containing your script
- Run this command:
chmod +x hello.sh
This command gives your script execute permissions.
Running Your Bash Script
Now you can run your script in three ways:
-
Using bash directly:
bash hello.sh
-
Using the full path:
/path/to/hello.sh
-
Using the current directory:
./hello.sh
Running Your Script as a Command
To run your script like any other command, you need to add its location to your PATH. Here's how:
-
Move your script to a directory in your PATH (like
/usr/local/bin
):sudo mv hello.sh /usr/local/bin/hello
-
Now you can run it from anywhere:
hello
Alternatively, you can add the script's directory to your PATH:
-
Open your
.bashrc
or.bash_profile
file:nano ~/.bashrc
-
Add this line at the end of the file:
export PATH="$PATH:/path/to/your/script/directory"
-
Save the file and reload it:
source ~/.bashrc
Now you can run your script as a command from anywhere.
Creating More Complex Scripts
Let's create a more useful script that tells us the current date and time:
#!/bin/bash
echo "Current date: $(date +"%Y-%m-%d")"
echo "Current time: $(date +"%H:%M:%S")"
Save this as datetime.sh
, make it executable, and move it to /usr/local/bin/datetime
.
Now you can run datetime
from anywhere in your terminal!
Variables in Bash Scripts
Variables make your scripts more flexible. Here's an example:
#!/bin/bash
name="World"
echo "Hello, $name!"
You can also accept input from the user:
#!/bin/bash
echo "What's your name?"
read name
echo "Hello, $name!"
Conditional Statements
Bash scripts can make decisions using if
statements:
#!/bin/bash
echo "Enter a number:"
read num
if [ $num -gt 10 ]
then
echo "The number is greater than 10"
else
echo "The number is less than or equal to 10"
fi
Loops in Bash Scripts
Loops help you repeat tasks. Here's a simple for
loop:
#!/bin/bash
for i in {1..5}
do
echo "Number: $i"
done
And a while
loop:
#!/bin/bash
count=1
while [ $count -le 5 ]
do
echo "Count: $count"
((count++))
done
Functions in Bash Scripts
Functions help organize your code:
#!/bin/bash
greet() {
echo "Hello, $1!"
}
greet "World"
greet "Bash Learner"
Command-Line Arguments
You can pass arguments to your script when you run it:
#!/bin/bash
echo "Script name: $0"
echo "First argument: $1"
echo "Second argument: $2"
echo "All arguments: $@"
Run this script with ./script.sh arg1 arg2 arg3
.
Error Handling
It's important to handle errors in your scripts:
#!/bin/bash
# Exit the script if any command fails
set -e
# Function to handle errors
handle_error() {
echo "An error occurred on line $1"
exit 1
}
# Set up error handling
trap 'handle_error $LINENO' ERR
# Your script commands here
Practical Example: Backup Script
Let's create a useful backup script:
#!/bin/bash
# Set the source and destination directories
source_dir="/path/to/source"
backup_dir="/path/to/backup"
# Create a timestamp
timestamp=$(date +"%Y%m%d_%H%M%S")
# Create the backup
backup_file="backup_$timestamp.tar.gz"
tar -czf "$backup_dir/$backup_file" "$source_dir"
echo "Backup created: $backup_file"
Save this as backup.sh
, make it executable, and move it to /usr/local/bin/backup
.
Now you can run backup
anytime to create a timestamped backup of your files!
Advanced Bash Scripting Techniques
Regular Expressions
Bash supports regular expressions for pattern matching:
#!/bin/bash
string="Hello, World!"
if [[ $string =~ ^Hello ]]
then
echo "String starts with 'Hello'"
fi
Process Substitution
You can use process substitution to pass the output of a command as a file:
#!/bin/bash
diff <(ls dir1) <(ls dir2)
Heredocs
Heredocs allow you to pass multiline strings to commands:
#!/bin/bash
cat << EOF > output.txt
This is line 1
This is line 2
EOF
Best Practices for Bash Scripting
- Always use the shebang line (
#!/bin/bash
) - Use meaningful variable names
- Comment your code
- Use functions for reusable code
- Handle errors and edge cases
- Use
set -e
to exit on errors - Use
set -u
to catch unset variables - Use
set -o pipefail
to catch pipeline errors - Use double quotes around variables to prevent word splitting
Debugging Bash Scripts
To debug your scripts, you can use the -x
option:
bash -x ./script.sh
This will print each command before it's executed.
You can also use the set -x
command within your script to enable debugging for specific sections:
#!/bin/bash
set -x # Turn on debugging
# Debugging-enabled section
set +x # Turn off debugging
Security Considerations
When writing bash scripts, keep these security tips in mind:
- Always validate and sanitize user input
- Use full paths for commands and files
- Be cautious when using
eval
or executing user-provided commands - Set appropriate permissions on your scripts
- Use
readonly
for constants to prevent accidental modification
Conclusion
Congratulations! You've learned how to create, run, and even turn your bash scripts into commands. You've also explored more advanced concepts like functions, loops, and error handling. This is just the beginning of your bash scripting journey. With these skills, you can automate tasks, manage your system, and boost your productivity.
Remember, the best way to learn is by doing. Try modifying the scripts in this guide, create your own, and most importantly, have fun with it! Bash scripting is a powerful tool that can make your life easier and your work more efficient.
Happy scripting!