HTTP Only Cookies vs. JWT Tokens: A Deep Dive
Choosing between HTTP Only Cookies and JWT Tokens for user authentication? This guide compares their security, ease of use, and scalability, helping you decide the best option for your project. Learn about best practices and how to combine both methods for enhanced security.
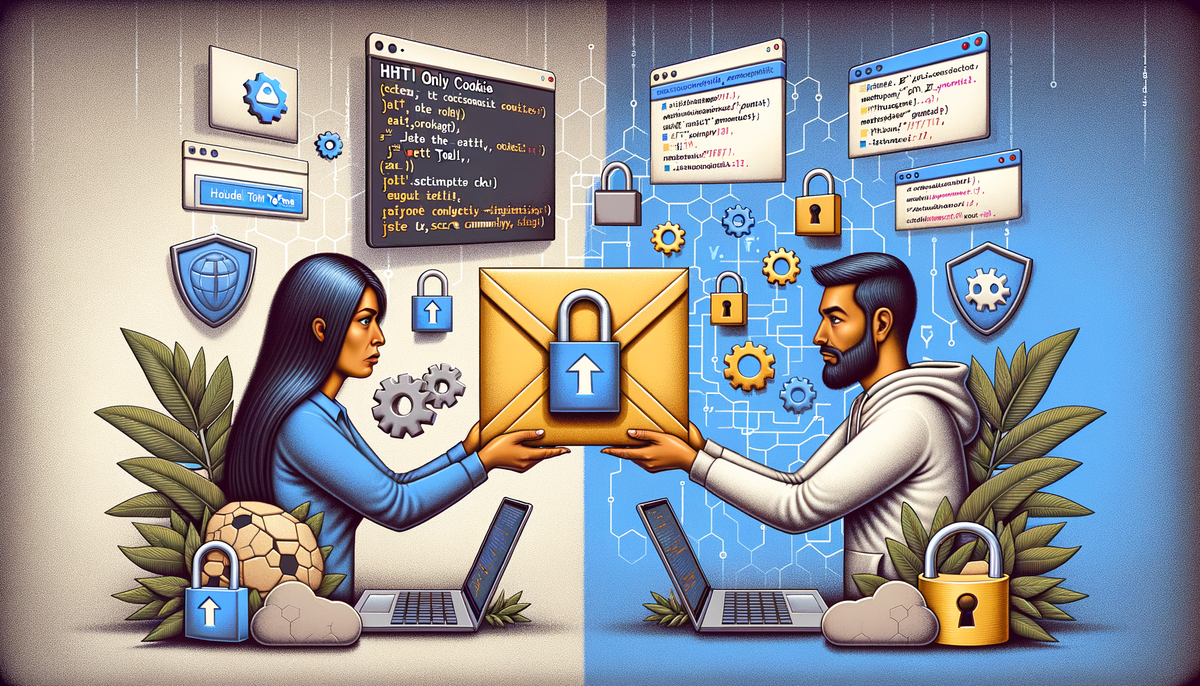
You're building a website or app, and you need a way to keep track of who's logged in. Two common options for this are HTTP Only Cookies and JWT Tokens. This guide will help you choose the best option for your project.
What are HTTP Only Cookies?
Imagine a tiny note you give to your friend, but you don't want anyone else to read it. This is like an HTTP Only Cookie. It's a small piece of information sent from a website to your computer, but only the website can read it—not other websites or your computer.
How to Make an HTTP Only Cookie
Let's say you're building a website using Node.js. To create an HTTP Only Cookie, you can use a special setting in your code.
// Node.js Example
res.cookie('session', '123456', { httpOnly: true, secure: true });
This code tells the website to create a cookie called "session" that only the website can read.
Example: Using HTTP Only Cookies in Express
Here's a complete example of how to use HTTP Only Cookies in Express.js, a popular framework for Node.js websites.
const express = require('express');
const app = express();
app.get('/login', (req, res) => {
// Create a unique session ID (could be more complex in real usage)
const sessionID = 'sessionId12345';
// Set the HTTP Only Cookie
res.cookie('session', sessionID, { httpOnly: true, secure: true });
res.send('Logged in successfully');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
This code creates a login page. When a user logs in, it gives them a unique session ID in an HTTP Only Cookie, so only the website can read it.
What are JWT Tokens?
Imagine a secret message you write to your friend, and you want to make sure no one else can read it. You use a special code to encrypt the message, and your friend uses the same code to decrypt it. This is like a JWT Token. It's a small piece of information that contains some data, like a user's ID, and is encrypted so only the website can read it.
How to Make a JWT Token
To make a JWT Token, you can use a special library. Here's an example in Node.js.
const jwt = require('jsonwebtoken');
const payload = { userId: 123 };
const secret = 'mySuperSecret';
const token = jwt.sign(payload, secret, { expiresIn: '1h' });
console.log(token);
This code creates a JWT Token that contains a user ID and will expire in one hour.
Example: Using JWT Tokens in Express
Here's a complete example of how to create and use JWT Tokens in Express.js.
const express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
const secret = 'mySuperSecret';
app.get('/login', (req, res) => {
const payload = { userId: 123 };
const token = jwt.sign(payload, secret, { expiresIn: '1h' });
// Send the token to the client
res.cookie('token', token, { httpOnly: true, secure: true });
res.send('Logged in with JWT');
});
app.get('/protected', (req, res) => {
const token = req.cookies.token;
if (!token) return res.sendStatus(401);
jwt.verify(token, secret, (err, decoded) => {
if (err) return res.sendStatus(403);
res.send(`Hello, user ${decoded.userId}`);
});
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
This code creates a login page and a protected page. When a user logs in, it gives them a JWT Token, which is stored in an HTTP Only Cookie. When the user tries to access the protected page, the website checks the JWT Token to verify their identity.
Comparing HTTP Only Cookies and JWT Tokens
Now, let's compare HTTP Only Cookies and JWT Tokens to see which one is best for you.
Security
- HTTP Only Cookies: Because only the website can read HTTP Only Cookies, they are safe from hackers who try to steal your information through your web browser.
- JWT Tokens: JWT Tokens can be safer if you store them in HTTP Only Cookies. However, if they are stored in other places, like your browser's local storage, they can be more vulnerable.
Ease of Use
- HTTP Only Cookies: HTTP Only Cookies are easy to use because the website automatically handles them.
- JWT Tokens: JWT Tokens require a bit more work because you need to manually add them to each request you send to the website.
Scalability
- HTTP Only Cookies: HTTP Only Cookies work well for traditional websites where the website handles all the user information.
- JWT Tokens: JWT Tokens are great for websites that use many different services, like microservices, because they are stateless and don't rely on the website to store user information.
Combining Both Methods
You can also use both methods together for even better security. You can store a JWT Token in an HTTP Only Cookie, and your website can then use the token to verify your identity.
const token = jwt.sign(payload, secret, { expiresIn: '1h' });
res.cookie('token', token, { httpOnly: true, secure: true });
In your client application, you can ensure that the token is sent with each request:
axios.defaults.withCredentials = true;
Best Practices
Keeping HTTP Only Cookies Safe
- Secure Connection: Always make sure your website uses a secure connection (HTTPS) to protect your data.
- SameSite Policy: Use the
SameSite
attribute to prevent hackers from using your cookies on other websites.
Keeping JWT Tokens Safe
- Verification: Always check that the JWT Token is valid before allowing access.
- Short Expiry Time: Make sure JWT Tokens expire after a short period of time to reduce the risk of being compromised.
- Secret Code: Keep the secret code used for encrypting JWT Tokens safe and secure.
Conclusion
Both HTTP Only Cookies and JWT Tokens have their advantages. HTTP Only Cookies offer excellent security, while JWT Tokens provide flexibility and scalability. Choose the method that best fits your project's needs. Always follow security best practices to protect your data.
Summary
HTTP Only Cookies are like secret notes that only the website can read, while JWT Tokens are like encrypted messages that can be shared between different services. Both methods can keep your user information safe, but HTTP Only Cookies are generally safer. You can even combine both methods for even better security. Remember to keep your data safe by following security best practices.