Migrating from Create React App to ViteJS: A Step-by-Step Guide
Supercharge your React development by migrating from Create React App to ViteJS. Learn why ViteJS is a game-changer and follow our step-by-step guide to enjoy faster builds, smaller bundles, and enhanced flexibility. Boost your productivity today! #ReactJS #ViteJS #WebDev
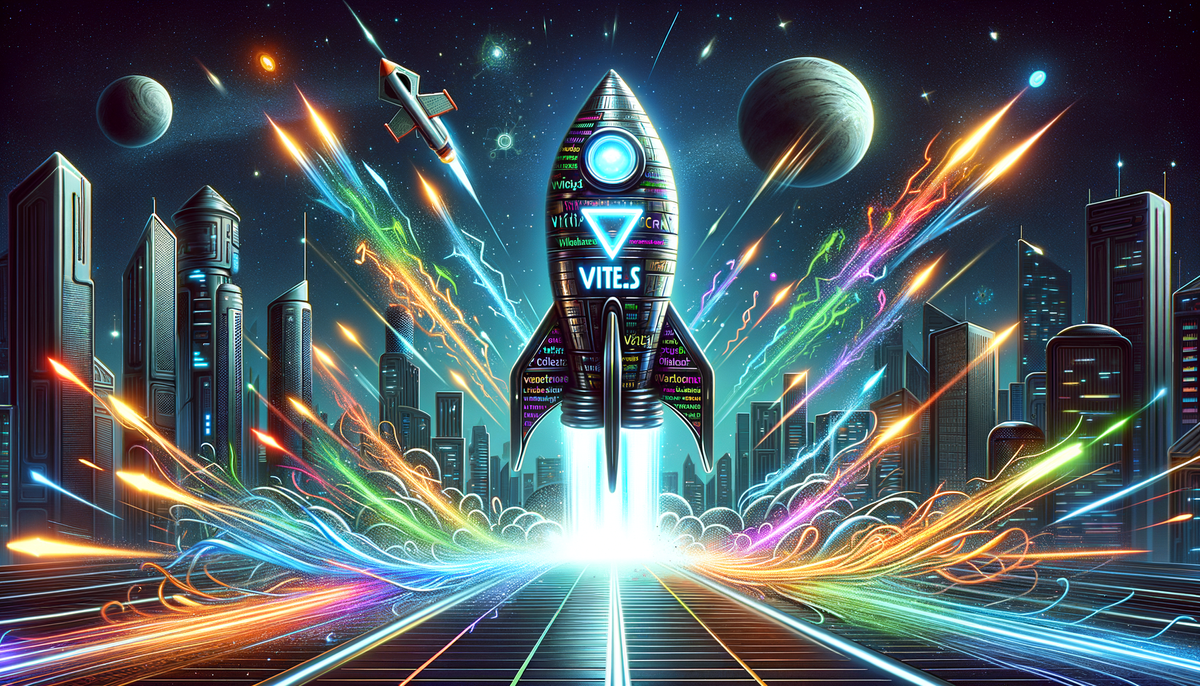
Are you tired of slow build times and sluggish hot reloads in your React project? It might be time to switch from Create React App (CRA) to ViteJS. This guide will walk you through the migration process, helping you boost your development productivity and improve your app's performance.
What is ViteJS?
ViteJS is a modern, lightning-fast build tool and development server. It leverages native ES modules in the browser, enabling instant server starts and rapid hot module replacement (HMR). ViteJS is designed to streamline your development workflow and optimize your application's performance.
Why Migrate from Create React App to ViteJS?
- Blazing Fast Development: ViteJS offers near-instant server starts and lightning-fast HMR, significantly speeding up your development process.
- Smaller Bundle Sizes: With its pre-bundling step, ViteJS optimizes dependencies, resulting in smaller bundle sizes and faster load times.
- Enhanced Flexibility: ViteJS provides a more configurable build system than CRA, allowing for greater customization.
- Modern Tooling: Embrace cutting-edge web development tools, including native ES modules and robust TypeScript support.
- Improved Performance: ViteJS's optimized build process can lead to better runtime performance for your application.
Step-by-Step Migration Guide
Step 1: Install ViteJS
Ensure you have Node.js installed, then run:
npm install -g vite
Step 2: Create a New ViteJS Project
Navigate to your desired directory and run:
npm create vite@latest my-vite-app -- --template react
Replace my-vite-app
with your project name.
Step 3: Copy Source Files
Transfer your CRA project files to the new ViteJS project:
- Move
src
directory contents to the ViteJSsrc
folder. - Copy static assets from
public
to the ViteJSpublic
folder.
Step 4: Update Import Paths
Adjust import paths in your source files. For example:
// Before (CRA)
import MyComponent from './components/MyComponent';
// After (ViteJS)
import MyComponent from './components/MyComponent';
Note: ViteJS doesn't require changing relative import paths, unlike the previous example suggested.
Step 5: Configure ViteJS
Create or modify vite.config.js
in your project root:
import { defineConfig } from 'vite';
import react from '@vitejs/plugin-react';
export default defineConfig({
plugins: [react()],
build: {
outDir: 'build',
},
resolve: {
alias: {
'@': '/src',
},
},
});
This configuration adds the React plugin, sets the build output directory, and creates an alias for easier imports.
Step 6: Update Package.json Scripts
Modify your package.json
scripts:
"scripts": {
"dev": "vite",
"build": "vite build",
"preview": "vite preview",
"test": "vitest"
}
Step 7: Install Dependencies
Run:
npm install
Step 8: Update Environment Variables
Rename .env
files to use the Vite prefix:
.env
to.env
.env.development
to.env.development
.env.production
to.env.production
Update environment variable usage in your code:
// Before (CRA)
process.env.REACT_APP_API_URL
// After (ViteJS)
import.meta.env.VITE_API_URL
Make sure to prefix your environment variables with VITE_
for them to be exposed to your application.
Step 9: Update Test Setup
If you're using Jest with CRA, you'll need to switch to Vitest, which integrates seamlessly with Vite:
-
Install Vitest:
npm install -D vitest
-
Update your test files to use Vitest syntax (which is very similar to Jest).
-
Create a
vitest.config.js
file in your project root:import { defineConfig } from 'vitest/config'; import react from '@vitejs/plugin-react'; export default defineConfig({ plugins: [react()], test: { environment: 'jsdom', globals: true, }, });
Step 10: Run the Development Server
Start your ViteJS development server:
npm run dev
Your app should now be running with ViteJS!
Tips and Tricks
- CSS Modules: ViteJS supports CSS Modules out of the box. Just name your CSS files with the
.module.css
extension. - Code Splitting: Use dynamic imports for automatic code splitting:
const MyComponent = lazy(() => import('./MyComponent'));
- Optimization: ViteJS automatically optimizes your dependencies. For further optimization, consider using the
build.rollupOptions
in your Vite config.
Conclusion
Migrating from Create React App to ViteJS can significantly enhance your development experience and application performance. This guide covers the essential steps, but remember to consult the ViteJS documentation for more advanced features and optimizations.
By embracing ViteJS, you're setting yourself up for faster development cycles, improved build times, and a more modern React development environment. Happy coding with ViteJS!