Multithreading in Go vs Rust: Performance and Efficiency Compared
Explore the performance and efficiency of multithreading in Go vs Rust. Learn about goroutines, channels, and Rust's safe concurrency model. Discover when to choose each language for your projects, and see real-world examples and benchmarks comparing their multithreading capabilities.
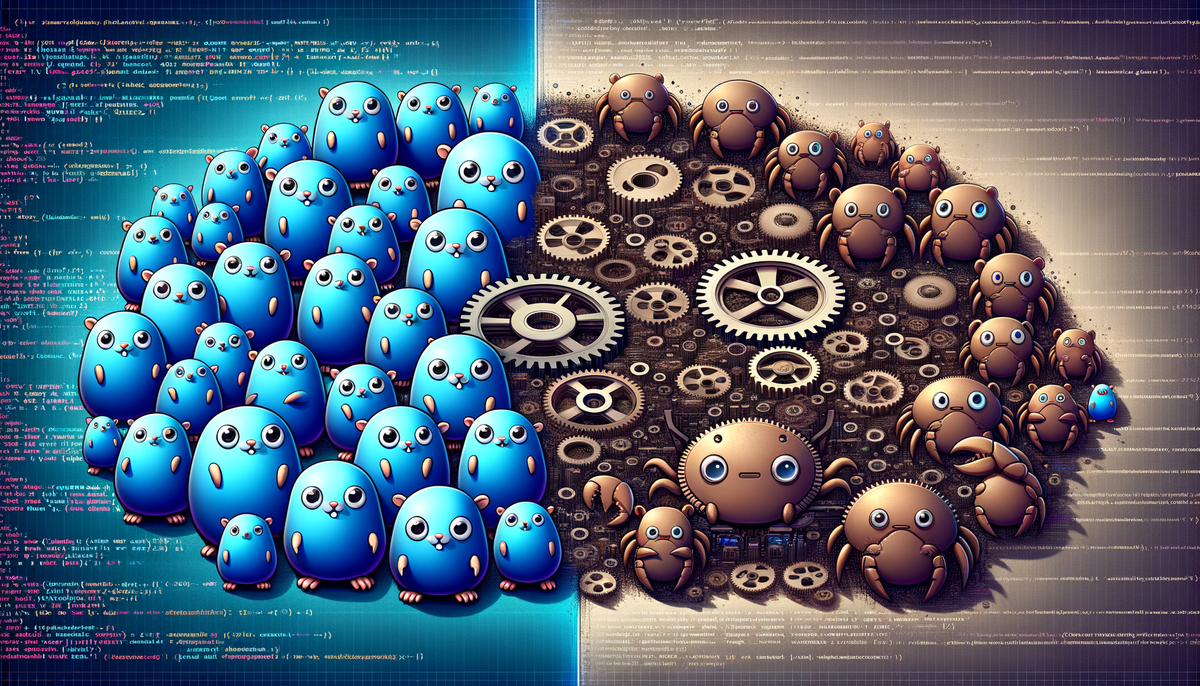
In today's world of fast computers and big data, we need our programs to work quickly. One way to make programs faster is by using multiple threads. Threads are like small workers that can do different tasks at the same time. Two popular programming languages, Go and Rust, are great at using threads. Let's compare how they do it and see which one might be better for your needs.
What is Multithreading?
Multithreading is like having many helpers work on a big project together. Instead of one person doing everything, many people can work on different parts at the same time. This makes the work get done faster.
In computer programs, threads are these helpers. They can do different jobs at the same time, making the program run faster.
Go's Approach to Multithreading
Go, also called Golang, makes multithreading easy. It uses something called "goroutines" and "channels" to help programmers write code that can do many things at once.
Goroutines
Goroutines are like light workers. They're easy to create and don't need much computer power. You can make thousands of goroutines without slowing down your computer.
Here's a simple example of how to use a goroutine in Go:
package main
import (
"fmt"
"time"
)
func sayHello() {
fmt.Println("Hello from goroutine!")
}
func main() {
go sayHello()
time.Sleep(time.Second)
fmt.Println("Main function")
}
In this example, we create a goroutine that prints "Hello from goroutine!". The main function continues running while the goroutine does its job.
Channels
Channels in Go are like pipes that let goroutines talk to each other. They help goroutines share information safely.
Here's an example of using channels:
package main
import "fmt"
func sum(s []int, c chan int) {
sum := 0
for _, v := range s {
sum += v
}
c <- sum
}
func main() {
s := []int{7, 2, 8, -9, 4, 0}
c := make(chan int)
go sum(s[:len(s)/2], c)
go sum(s[len(s)/2:], c)
x, y := <-c, <-c
fmt.Println(x, y, x+y)
}
This program splits a list of numbers, adds them up in two goroutines, and then combines the results using a channel.
Rust's Approach to Multithreading
Rust takes a different approach to multithreading. It focuses on safety and preventing errors that can happen when many threads work together.
Threads in Rust
Rust uses a standard library called std::thread
for creating and managing threads. These threads are more like traditional operating system threads.
Here's a simple example of creating a thread in Rust:
use std::thread;
use std::time::Duration;
fn main() {
thread::spawn(|| {
println!("Hello from a thread!");
});
thread::sleep(Duration::from_millis(1));
println!("Main thread");
}
This code creates a new thread that prints a message, similar to our Go example.
Safe Concurrency with Ownership and Borrowing
Rust uses a system of ownership and borrowing to make sure threads don't accidentally mess up each other's data. This can make writing multithreaded code a bit harder, but it prevents many common errors.
Here's an example of sharing data between threads in Rust:
use std::thread;
use std::sync::{Arc, Mutex};
fn main() {
let counter = Arc::new(Mutex::new(0));
let mut handles = vec![];
for _ in 0..10 {
let counter = Arc::clone(&counter);
let handle = thread::spawn(move || {
let mut num = counter.lock().unwrap();
*num += 1;
});
handles.push(handle);
}
for handle in handles {
handle.join().unwrap();
}
println!("Result: {}", *counter.lock().unwrap());
}
This program creates 10 threads that all add to the same counter. The Arc
and Mutex
types make sure this is done safely.
Performance Comparison
When it comes to speed and efficiency, both Go and Rust perform well, but they have different strengths.
Go's Performance
- Quick Start: Go programs start up very fast.
- Easy Concurrency: Creating goroutines is simple and doesn't use much memory.
- Garbage Collection: Go automatically cleans up memory, which can sometimes cause short pauses.
Rust's Performance
- Raw Speed: Rust can be very fast, often as fast as C.
- Memory Efficiency: Rust doesn't need garbage collection, so it can use less memory.
- Predictable Performance: Rust's performance is more consistent because it doesn't have garbage collection pauses.
Efficiency Comparison
Efficiency is about how well a language uses computer resources like memory and CPU time.
Go's Efficiency
- Memory Usage: Go uses more memory because of its garbage collector, but it's easier to write programs that don't leak memory.
- CPU Usage: Go is good at using multiple CPU cores with its goroutines.
Rust's Efficiency
- Memory Usage: Rust uses less memory and gives programmers more control over memory use.
- CPU Usage: Rust can use CPU very efficiently, but it requires more careful programming.
When to Choose Go for Multithreading
Go might be the better choice when:
- You need to write concurrent programs quickly.
- Your program needs to handle many connections at once (like a web server).
- You want a language that's easy to learn and use.
- You're building microservices or distributed systems.
When to Choose Rust for Multithreading
Rust might be the better choice when:
- You need the highest possible performance.
- Memory safety is critical (like in systems programming).
- You want fine-grained control over system resources.
- You're building programs that can't afford any pauses (like game engines or audio processing).
Real-World Examples
Let's look at some real-world examples where Go and Rust's multithreading capabilities shine:
Go Example: Web Server
Go is great for building web servers that can handle many requests at once. Here's a simple example:
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, %s!", r.URL.Path[1:])
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This server can handle many connections simultaneously, thanks to Go's efficient goroutines.
Rust Example: Parallel Data Processing
Rust excels at processing large amounts of data in parallel. Here's a simple example:
use rayon::prelude::*;
fn main() {
let numbers: Vec<i32> = (1..1_000_000).collect();
let sum: i32 = numbers.par_iter().sum();
println!("Sum is {}", sum);
}
This code uses the Rayon library to parallelize the sum calculation, making it much faster on multi-core systems.
Benchmark Comparison
To give you a concrete idea of performance differences, here's a simple benchmark comparing Go and Rust for a common multithreading task: calculating prime numbers.
Go version:
package main
import (
"fmt"
"math"
"sync"
"time"
)
func isPrime(n int) bool {
if n <= 1 {
return false
}
for i := 2; i <= int(math.Sqrt(float64(n))); i++ {
if n%i == 0 {
return false
}
}
return true
}
func main() {
start := time.Now()
var wg sync.WaitGroup
for i := 2; i < 1000000; i++ {
wg.Add(1)
go func(n int) {
defer wg.Done()
if isPrime(n) {
// fmt.Printf("%d is prime\n", n)
}
}(i)
}
wg.Wait()
elapsed := time.Since(start)
fmt.Printf("Time taken: %s\n", elapsed)
}
Rust version:
use std::time::Instant;
use rayon::prelude::*;
fn is_prime(n: u32) -> bool {
if n <= 1 {
return false;
}
for i in 2..=(f64::from(n).sqrt() as u32) {
if n % i == 0 {
return false;
}
}
true
}
fn main() {
let start = Instant::now();
(2..1000000).into_par_iter().for_each(|n| {
if is_prime(n) {
// println!("{} is prime", n);
}
});
let duration = start.elapsed();
println!("Time taken: {:?}", duration);
}
On a typical modern computer, you might find that the Rust version is slightly faster, but Go's version is easier to write and understand.
Conclusion
Both Go and Rust are great for multithreading, but they suit different needs:
- Go makes it easy to write concurrent programs quickly. It's great for web services and when you need to handle lots of tasks at once.
- Rust gives you more control and safety. It's perfect for programs that need to be very fast and use memory efficiently.
When choosing between Go and Rust for multithreading, think about what your program needs to do. If you want something quick to write and easy to understand, Go might be best. If you need the fastest possible program and don't mind spending more time writing it, Rust could be the way to go.
Remember, the best language is the one that solves your problem most effectively. Both Go and Rust are powerful tools for multithreading, each with its own strengths. Consider your project requirements, team expertise, and performance needs when making your choice.