MySQL vs PostgreSQL: A Comprehensive Comparison
Choosing the right database is crucial. This guide compares MySQL and PostgreSQL, highlighting key differences in performance, features, data integrity, and extensibility. Learn which is best for your project, whether it's a simple web app or a complex enterprise system.
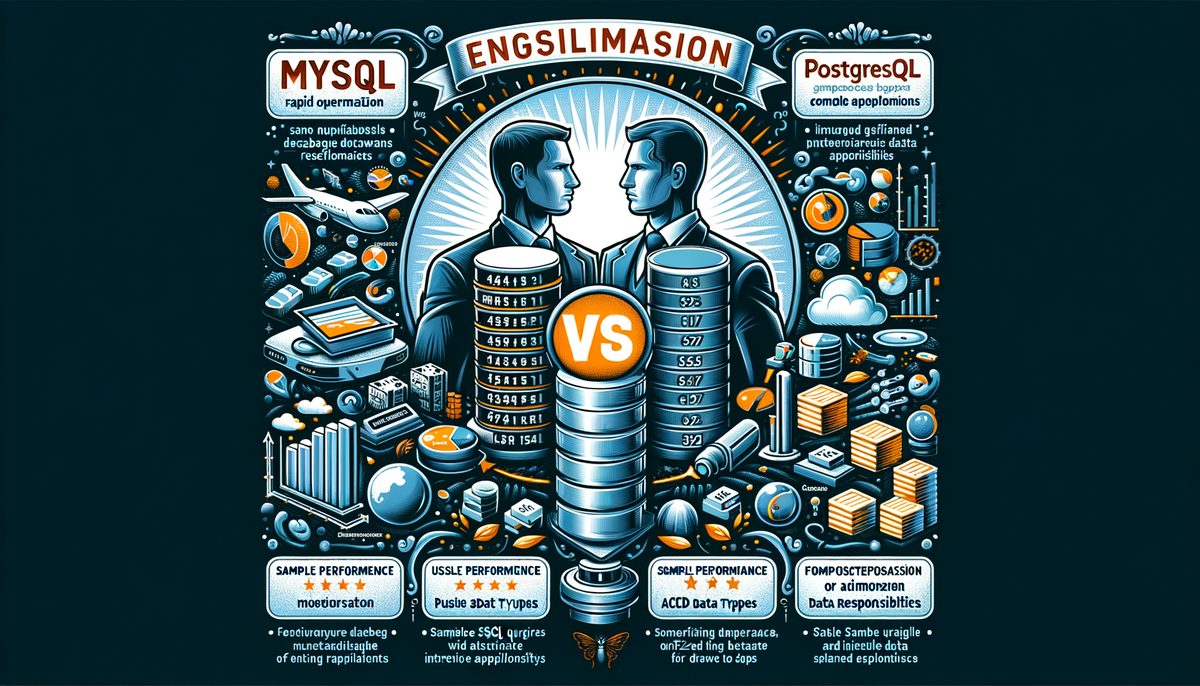
Choosing the right database management system (DBMS) is a crucial decision for any software project. This guide compares two popular open-source relational database management systems (RDBMS): MySQL and PostgreSQL. By understanding their key differences, you'll be able to select the best fit for your needs, whether it's a simple web application or a complex, enterprise-level system.
What is MySQL?
MySQL is a widely used, open-source RDBMS known for its speed and ease of use. It's a popular choice for web applications, particularly those that need fast data retrieval. Some of MySQL's key features include:
- Speed: MySQL excels at handling read-heavy operations, meaning it quickly retrieves data.
- Ease of Use: Its simple syntax and user-friendly interface make it great for beginners.
- Replication: MySQL's replication capabilities allow for high availability and scalability, ensuring your data is always accessible and your application can handle growing user demands.
Example: Basic MySQL Query
-- Create a database
CREATE DATABASE sample_db;
-- Select the database to use
USE sample_db;
-- Create a table called 'users'
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100)
);
-- Insert data into the 'users' table
INSERT INTO users (name, email) VALUES ('John Doe', 'john.doe@example.com');
-- Retrieve all data from the 'users' table
SELECT * FROM users;
What is PostgreSQL?
PostgreSQL is another open-source RDBMS that emphasizes data integrity, advanced features, and powerful querying capabilities. It's often preferred for applications requiring complex data manipulation and robust transactional integrity. Some of PostgreSQL's key strengths include:
- Advanced Features: PostgreSQL supports a wide array of data types, including JSON, geographic data (PostGIS), and custom data types. It also offers advanced features like full-text search and user-defined functions.
- ACID Compliance: PostgreSQL strictly adheres to ACID properties (Atomicity, Consistency, Isolation, Durability), ensuring reliable transactions and consistent data even in complex scenarios.
- PostGIS: An extension that allows for managing geographic data, making PostgreSQL ideal for mapping and location-based services.
Example: Basic PostgreSQL Query
-- Create a database
CREATE DATABASE sample_db;
-- Connect to the database
\c sample_db
-- Create a table called 'users'
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100)
);
-- Insert data into the 'users' table
INSERT INTO users (name, email) VALUES ('John Doe', 'john.doe@example.com');
-- Retrieve all data from the 'users' table
SELECT * FROM users;
Key Differences: MySQL vs. PostgreSQL
1. Performance
- MySQL: Typically performs well with read-heavy workloads but may struggle with complex write operations or queries involving large amounts of data.
- PostgreSQL: Known for its robust performance handling complex queries and write operations. It often excels in scenarios involving complex data structures or intensive data manipulation.
Example: Performance Tests
MySQL (Web application using simple queries):
-- Retrieve products from a specific category
SELECT * FROM products WHERE category = 'Electronics';
-- Add a new order
INSERT INTO orders (product_id, user_id, quantity) VALUES (1, 2, 3);
PostgreSQL (Web application using complex queries and data types):
-- Retrieve the name of users living in New York, using JSONB data type
SELECT data->>'name' AS name
FROM users
WHERE data->>'city' = 'New York';
-- Insert a transaction with metadata, using JSONB data type
INSERT INTO transactions (user_id, amount, metadata)
VALUES (1, 100.50, '{"type":"payment", "method":"credit"}');
2. Features
- MySQL: Offers basic data types and features, making it easier to learn and use.
- PostgreSQL: Provides a rich set of advanced features, including various data types, indexes, full-text search capabilities, and support for custom functions.
Example: Feature Usage
MySQL (Simple full-text search):
-- Create a full-text index on the 'content' column of the 'articles' table
CREATE FULLTEXT INDEX ft_index ON articles(content);
PostgreSQL (More sophisticated full-text search with GIN index):
-- Create a GIN index for full-text search on the 'content' column
CREATE INDEX gin_idx ON articles USING GIN(to_tsvector('english', content));
3. Data Integrity
- MySQL: Suitable for applications where data integrity is not the primary concern.
- PostgreSQL: Strongly adheres to ACID principles, making it a reliable choice for applications where data accuracy and consistency are paramount.
Example: Data Integrity
MySQL (Potential for transaction inconsistencies under high load):
-- MySQL transaction example
START TRANSACTION;
INSERT INTO account (name, balance) VALUES ('Alice', 1000);
UPDATE account SET balance = balance - 500 WHERE name = 'Alice';
COMMIT;
PostgreSQL (Ensuring strict ACID compliance):
-- PostgreSQL transaction example
BEGIN;
INSERT INTO account (name, balance) VALUES ('Alice', 1000);
UPDATE account SET balance = balance - 500 WHERE name = 'Alice';
COMMIT;
4. Extensibility
- MySQL: Limited support for custom extensions.
- PostgreSQL: Highly extensible through custom procedures, plugins, and support for other programming languages.
Example: Extensibility
MySQL (Functional, but limited customizability):
-- MySQL stored procedure
DELIMITER //
CREATE PROCEDURE addOrder(IN userId INT, IN productId INT, IN quantity INT)
BEGIN
INSERT INTO orders (user_id, product_id, quantity) VALUES (userId, productId, quantity);
END//
DELIMITER ;
PostgreSQL (Rich custom functions using PL/pgSQL):
-- PostgreSQL custom function using PL/pgSQL
CREATE FUNCTION add_order(user_id INT, product_id INT, quantity INT) RETURNS VOID AS $$
BEGIN
INSERT INTO orders (user_id, product_id, quantity) VALUES (user_id, product_id, quantity);
END;
$$ LANGUAGE plpgsql;
When to Use MySQL
- Web Applications: Ideal for websites and content management systems like WordPress that require fast data retrieval and simple database structures.
- Simple Applications: A good choice for projects where speed and ease of use are priorities over advanced features.
- Ease of Use: A great starting point for beginners due to its straightforward setup and user-friendly interface.
Example: Web Application in MySQL
-- MySQL for a blog website
CREATE TABLE posts (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255),
content TEXT,
author VARCHAR(100)
);
INSERT INTO posts (title, content, author) VALUES ('First Post', 'This is my first blog post!', 'Admin');
SELECT * FROM posts;
When to Use PostgreSQL
- Complex Queries: A powerful option for applications involving complex data manipulation, data analysis, or large-scale data processing.
- Scalability: A robust choice for applications that need to handle growing data volumes and user demands.
- Custom Features: Well-suited for applications requiring advanced data types, full-text search, or custom functions for specific business logic.
Example: Complex Application in PostgreSQL
-- PostgreSQL for an e-commerce platform
CREATE TABLE products (
id SERIAL PRIMARY KEY,
name VARCHAR(255),
description TEXT,
price NUMERIC
);
CREATE TABLE customers (
id SERIAL PRIMARY KEY,
name VARCHAR(255),
email VARCHAR(255),
metadata JSONB
);
INSERT INTO products (name, description, price) VALUES ('Laptop', 'High-end gaming laptop', 1200.99);
INSERT INTO customers (name, email, metadata)
VALUES ('John Doe', 'john.doe@example.com', '{"loyalty_points": 150, "preferred_category": "electronics"}');
SELECT * FROM products;
SELECT * FROM customers WHERE metadata->>'loyalty_points' > '100';
Conclusion
Choosing between MySQL and PostgreSQL depends on your specific application requirements and priorities. For simpler web applications and projects requiring fast data retrieval, MySQL can be a good choice. However, for complex, data-intensive applications, applications requiring strict data integrity, or those that need to scale over time, PostgreSQL's advanced features and performance capabilities make it a strong contender.
By carefully considering your project's needs, you can choose the database management system that best aligns with your goals and ensures the success of your application.