NASA's Power of Ten: Writing Space-Proof Code
Explore NASA's "Power of Ten" coding rules for space missions! Learn how these principles can improve your code, from rocket launches to everyday apps. Discover practical examples and apply NASA's standards to write cleaner, more reliable software today.
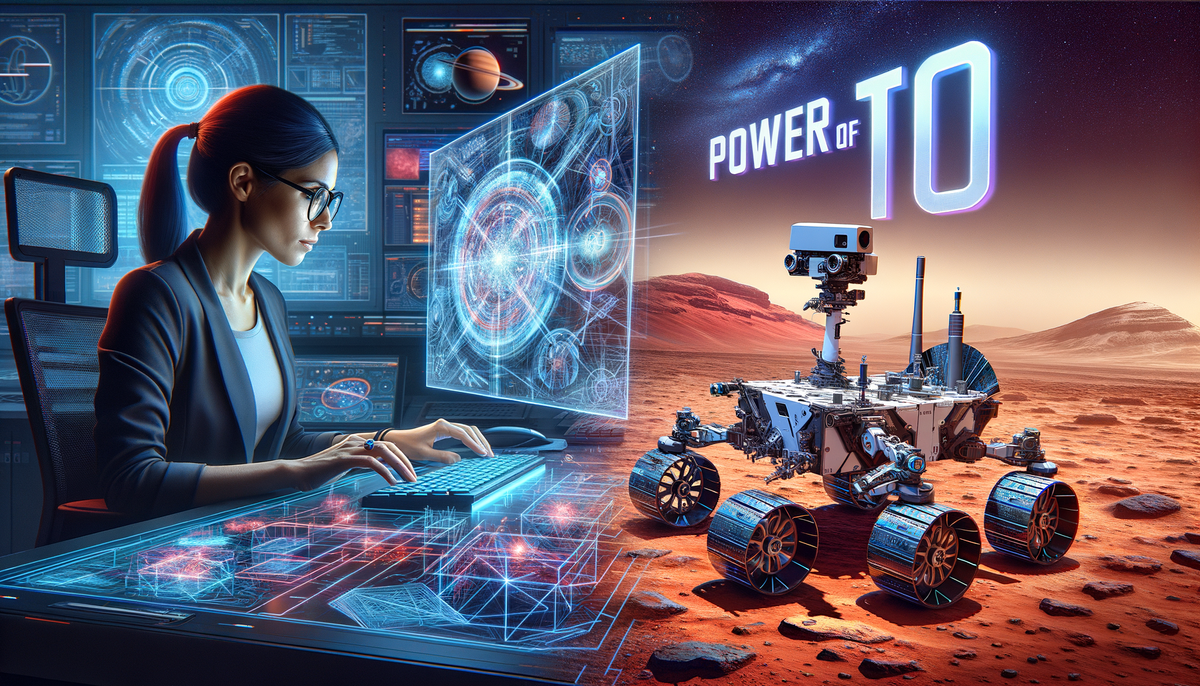
When it comes to coding for space missions, there's no room for error. NASA, the world's leading space agency, has developed a set of rules called the "Power of Ten" to ensure their software is as reliable as possible. Let's dive into these rules and see how they can help us write better code, whether we're sending rockets to Mars or building apps here on Earth.
What is the Power of Ten?
The Power of Ten is a set of coding rules created by Gerard J. Holzmann, a computer scientist at NASA's Jet Propulsion Laboratory. These rules are designed to make code simpler, more reliable, and easier to check for errors. They're not just for NASA - any programmer can use them to improve their code!
Here are the ten rules:
- No function should be longer than 50 lines of code
- Don't use more than one or two levels of loop nesting
- Don't use goto statements
- Don't use direct or indirect recursion
- Don't use more than one exit point from a loop or function
- Don't use dynamic memory allocation after initialization
- Check the return value of all non-void functions
- Use a minimum of two runtime assertions per function
- Don't use the preprocessor
- Limit pointer use to a single dereference
Let's break these down and see how we can apply them to our own coding practices.
Rule 1: Keep Functions Short
NASA says no function should be longer than 50 lines. Why? Short functions are easier to understand, test, and debug. Here's an example of how we might split a long function:
# Instead of this:
def do_everything():
# 100 lines of code doing many things
# Do this:
def prepare_data():
# 25 lines of code
def process_data():
# 25 lines of code
def analyze_results():
# 25 lines of code
def main():
prepare_data()
process_data()
analyze_results()
Rule 2: Limit Loop Nesting
Nested loops can be confusing and hard to follow. NASA says to use no more than one or two levels of loop nesting. Here's how we can improve deeply nested loops:
# Instead of this:
for i in range(10):
for j in range(10):
for k in range(10):
# Do something
# Do this:
def process_item(i, j, k):
# Do something
for i in range(10):
for j in range(10):
process_item_list(i, j)
def process_item_list(i, j):
for k in range(10):
process_item(i, j, k)
Rule 3: No Goto Statements
Goto statements can make code hard to follow. NASA says to avoid them completely. Most modern languages discourage or don't even allow goto statements, so this rule is easier to follow today.
Rule 4: Avoid Recursion
Recursion can be elegant, but it's also risky. It can lead to stack overflows and can be hard to reason about. NASA prefers iterative solutions:
# Instead of this:
def factorial(n):
if n == 0:
return 1
return n * factorial(n-1)
# Do this:
def factorial(n):
result = 1
for i in range(1, n+1):
result *= i
return result
Rule 5: One Exit Point
Having multiple return statements in a function can make it hard to understand all the ways a function might end. NASA recommends using a single exit point:
# Instead of this:
def check_value(x):
if x < 0:
return "Negative"
if x == 0:
return "Zero"
return "Positive"
# Do this:
def check_value(x):
result = "Positive" # Default case
if x < 0:
result = "Negative"
elif x == 0:
result = "Zero"
return result
Rule 6: Careful Memory Management
Dynamic memory allocation can lead to memory leaks and fragmentation. NASA recommends avoiding it after initialization. In languages with garbage collection, this is less of an issue, but it's still good to be mindful of memory use.
Rule 7: Check Return Values
Always check the return values of functions to catch and handle errors:
result = some_function()
if result is None:
# Handle the error
else:
# Proceed with the result
Rule 8: Use Runtime Assertions
Assertions help catch bugs early. NASA recommends at least two per function:
def calculate_rocket_speed(distance, time):
assert distance > 0, "Distance must be positive"
assert time > 0, "Time must be positive"
speed = distance / time
assert speed > 0, "Speed must be positive"
return speed
Rule 9: Avoid the Preprocessor
This rule is specific to C/C++, where the preprocessor can make code hard to understand. In other languages, we can interpret this as "avoid magic numbers and complex macros."
Rule 10: Limit Pointer Use
Again, this is more relevant to C/C++. In higher-level languages, we can interpret this as "be careful with references and complex data structures."
Applying Power of Ten in Your Projects
You don't need to be writing code for a Mars rover to benefit from these rules. Here's how you can start using them in your own projects:
- Review your functions and break long ones into smaller, focused parts.
- Simplify your loops and avoid deep nesting.
- Use iterative solutions instead of recursive ones where possible.
- Implement error checking for all function returns.
- Add assertions to catch impossible conditions early.
- Be mindful of memory usage, even in garbage-collected languages.
Real-World Impact of NASA's Coding Standards
NASA's coding standards have had a significant impact on mission success rates. For example:
- The Mars Curiosity Rover, which has been operational since 2012, uses software developed with these principles.
- The New Horizons mission to Pluto, which completed its flyby in 2015, relied on error-free code to function billions of miles from Earth.
These successes demonstrate the effectiveness of NASA's approach to software engineering in high-stakes environments.
Beyond Space: Applying NASA's Standards to Everyday Software
While NASA's standards were developed for space missions, they've found applications in other critical systems:
- Aviation software uses similar principles to ensure safety in commercial flights.
- Medical device software adopts strict coding standards to protect patient lives.
- Financial systems use rigorous coding practices to maintain data integrity and security.
By following these guidelines, you'll write cleaner, more reliable code that's easier to maintain and less prone to bugs. While you might not be sending your code to space, you'll still benefit from NASA's rigorous standards.
Remember, the goal of the Power of Ten is to make code simpler and more reliable. It's not about following rules blindly, but about understanding the principles behind them and applying them thoughtfully to your work.
So the next time you sit down to code, think like a NASA engineer. Your software might not control a spacecraft, but it can still benefit from being space-proof!