Profiling and Debugging Golang Memory Leaks: Understanding Heap and Stack Usage
Discover how to find and fix memory leaks in Go! Learn about heap and stack usage, use powerful tools like pprof and go-torch, and explore common causes of leaks. This guide provides practical tips and real-world examples to help you write efficient, leak-free Go programs.
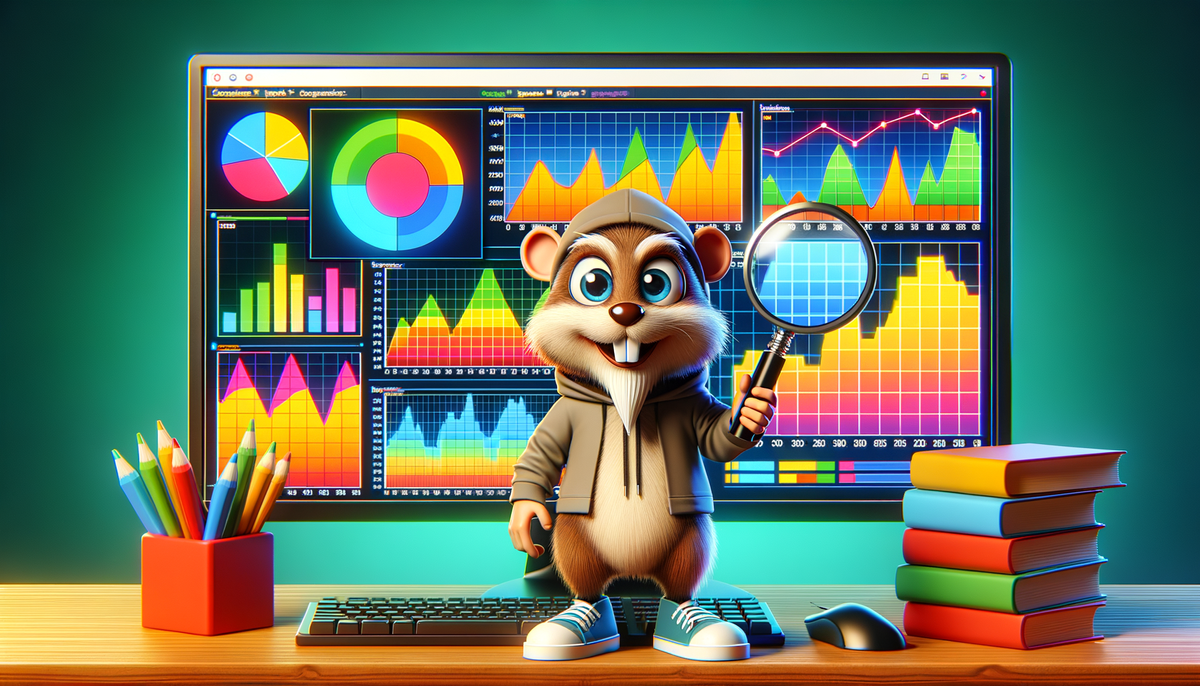
Memory leaks in Go programs can be tricky to find and fix. But don't worry! This guide will help you understand, detect, and solve memory leaks in your Go code. We'll explore tools, techniques, and best practices to keep your Go programs running smoothly.
What is a Memory Leak?
A memory leak happens when a program keeps using more and more memory over time, even when it doesn't need to. It's like forgetting to turn off the water faucet - the sink keeps filling up!
In Go, the garbage collector usually cleans up unused memory. But sometimes, it can't tell that some memory is no longer needed. That's when leaks happen.
Why Memory Leaks Matter
Memory leaks can cause big problems:
- Your program slows down
- It uses up all your computer's memory
- The program might crash
- Users get frustrated with slow or crashing apps
That's why it's important to find and fix memory leaks early!
Go's Memory Model: Stack and Heap
To understand memory leaks, let's look at how Go uses memory:
-
Stack:
- Fast and automatic
- Stores local variables
- Cleaned up when a function ends
-
Heap:
- Stores larger or longer-living data
- Managed by the garbage collector
- Where most memory leaks happen
Tools for Finding Memory Leaks
Go comes with great tools to help you find memory leaks. Let's explore them:
1. pprof
pprof
is Go's built-in profiling tool. It's like a detective for your code's memory use.
How to use pprof:
-
Add this to your code:
import _ "net/http/pprof" func main() { go func() { log.Println(http.ListenAndServe("localhost:6060", nil)) }() // Your main code here }
-
Run your program and then use this command:
go tool pprof http://localhost:6060/debug/pprof/heap
-
In the pprof console, type
top
to see the biggest memory users.
2. go-torch
go-torch
makes pretty flame graphs from pprof data. It's like a colorful map of your memory use.
To use go-torch:
-
Install it:
go get -u github.com/uber/go-torch
-
Run it:
go-torch -u http://localhost:6060/debug/pprof/heap
This creates a colorful graph showing where memory is being used.
3. runtime/debug package
The runtime/debug
package provides additional tools for debugging memory issues:
import "runtime/debug"
// Force garbage collection
debug.FreeOSMemory()
// Print stack traces of all goroutines
debug.PrintStack()
Common Causes of Memory Leaks in Go
Now, let's look at some common reasons for memory leaks:
-
Forgetting to Close Things
Always close files, network connections, and other resources when you're done.
Good example:
file, err := os.Open("bigfile.txt") if err != nil { log.Fatal(err) } defer file.Close() // This makes sure the file closes when we're done
-
Keeping Pointers to Big Things You Don't Need
If you keep pointing to data you don't need, Go can't clean it up.
Bad example:
var keeper []int func leakyFunc() { bigSlice := make([]int, 1000000) keeper = bigSlice[:1] // Oops! This keeps all million numbers in memory }
Good example:
var keeper []int func fixedFunc() { bigSlice := make([]int, 1000000) keeper = make([]int, 1) copy(keeper, bigSlice[:1]) // This only keeps one number }
-
Goroutine Leaks
If you start a goroutine and forget to stop it, it can keep using memory forever.
Bad example:
func leakyServer() { for { go handleRequest() // This keeps making new goroutines forever! } }
Good example:
func fixedServer(quit chan struct{}) { for { select { case <-quit: return default: go handleRequest() } } }
Step-by-Step Guide to Debug a Memory Leak
- Run your program with pprof
- Take a heap snapshot:
go tool pprof http://localhost:6060/debug/pprof/heap
- Look at the top memory users:
Typetop
in pprof to see which parts use the most memory. - Check suspicious functions:
Uselist functionName
in pprof to see the code of memory-hungry functions. - Look for leak patterns:
Check for unclosed resources, kept references, or runaway goroutines. - Fix the leak and test again
Best Practices to Avoid Memory Leaks
- Always close resources (files, connections, etc.)
- Be careful with global variables
- Use
defer
to make sure cleanup happens - Be mindful of how long goroutines live
- Check your program's memory use regularly
- Use tools like
go vet
andgolangci-lint
to find potential problems - Implement proper error handling to avoid resource leaks
- Use sync.Pool for frequently allocated and deallocated objects
Real-World Example: Fixing a Memory Leak
Let's look at a real example of finding and fixing a memory leak:
package main
import (
"fmt"
"net/http"
_ "net/http/pprof"
"time"
)
var leakySlice []string
func main() {
go func() {
fmt.Println(http.ListenAndServe("localhost:6060", nil))
}()
for {
leakyFunction()
time.Sleep(time.Second)
}
}
func leakyFunction() {
hugeString := string(make([]byte, 1024*1024)) // 1MB string
leakySlice = append(leakySlice, hugeString)
}
This program has a memory leak. It keeps adding big strings to leakySlice
without ever removing them.
To fix it, we can change leakyFunction
:
func fixedFunction() {
hugeString := string(make([]byte, 1024*1024))
if len(leakySlice) > 10 {
leakySlice = leakySlice[1:] // Remove the oldest string
}
leakySlice = append(leakySlice, hugeString)
}
Now, the slice never grows beyond 10 elements, preventing the memory leak.
Advanced Techniques
-
Continuous Profiling: Set up continuous profiling in production to catch memory leaks early.
-
Custom Memory Allocators: For performance-critical applications, consider implementing custom memory allocators.
-
Memory Pooling: Use object pools to reduce allocation and deallocation overhead.
-
Escape Analysis: Understand and leverage Go's escape analysis to optimize stack vs heap allocation.
Conclusion
Finding and fixing memory leaks in Go can be challenging, but with the right tools and knowledge, you can keep your programs running smoothly. Remember to:
- Use pprof and go-torch to find memory problems
- Look for common leak patterns
- Always clean up resources
- Be careful with long-living data and goroutines
- Regularly check your program's memory use
With practice, you'll get better at spotting and fixing memory leaks. Keep coding, keep learning, and enjoy building efficient Go programs!