Real-World Applications of Private-Public Key Cryptography: Securing Your Digital Life
Discover how private-public key cryptography secures your online life! Learn about its use in e-commerce, digital signatures, and secure communication. Explore Python code examples to understand how this technology keeps your information safe.
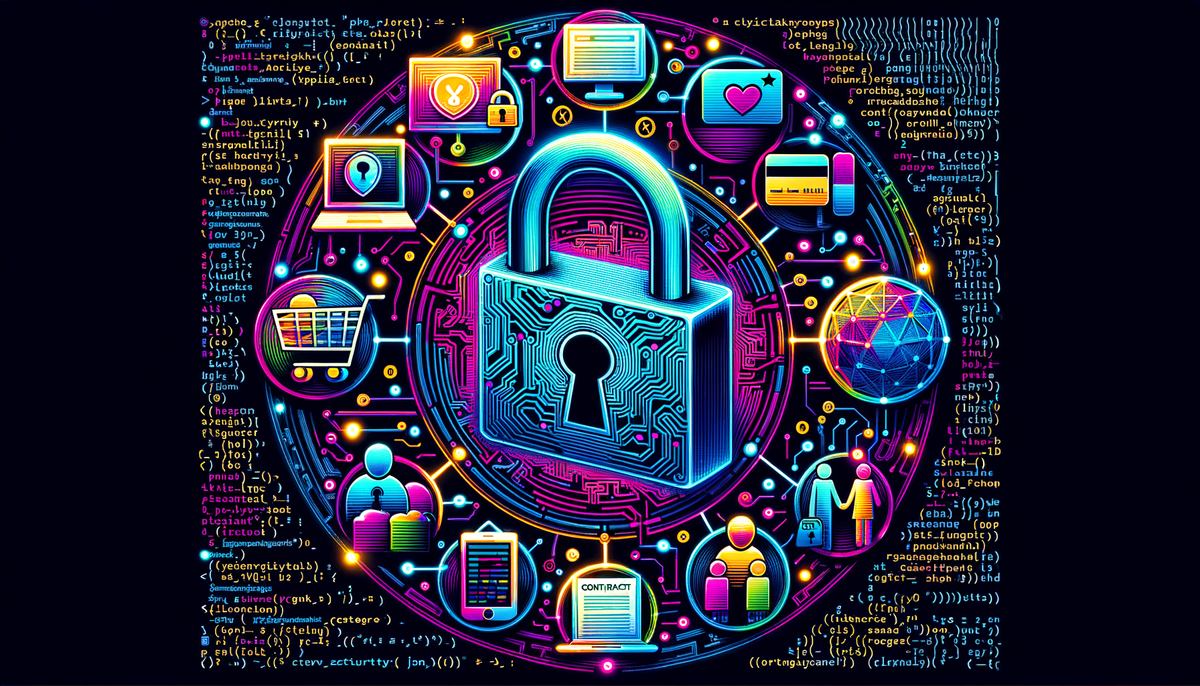
Introduction
Imagine you want to send a secret message to a friend. You write it down, but you don't want anyone else to read it. You could use a special code, but what if someone finds out the code? That's where private-public key cryptography comes in! It's like a magic lock and key. You have a private key that only you know, and a public key that you share with everyone. Only the private key can unlock the message that was locked with the public key. It's super safe and used in lots of ways to protect our online information. In this article, we'll explore how it works and how it keeps our online lives secure.
E-Commerce: Shopping Safely Online
How It Works
When you buy things online, you want to make sure your credit card information is safe. That's where private-public key cryptography comes in. The online store has a public key that everyone can see. When you enter your credit card details, the website uses the public key to encrypt it, turning the information into a scrambled code. Only the website can unlock it using their secret private key. It's like putting your information in a locked box that only the store can open.
Example: Python Code
Let's imagine you're an online store. You have a public key and a private key.
# Example of encrypting using a public key in Python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
# Generate public/private key pair
key_pair = RSA.generate(2048)
public_key = key_pair.publickey()
private_key = key_pair
message = b'Your credit card details'
# Encrypt message with public key
cipher = PKCS1_OAEP.new(public_key)
ciphertext = cipher.encrypt(message)
# Decrypt message with private key
cipher = PKCS1_OAEP.new(private_key)
plaintext = cipher.decrypt(ciphertext)
print(plaintext.decode()) # Output: Your credit card details
In this example, the public_key
is used to encrypt your credit card information. Only the private_key
can decrypt the information, keeping it safe during the transaction.
Digital Signatures: Signing Documents with Confidence
How It Works
Sometimes, we need to know that a document is real and hasn't been changed. That's where digital signatures come in! It's like putting your signature on a paper document, but using technology instead.
Let's say you want to send a contract to someone. You use your private key to create a digital signature. This signature is like a fingerprint, unique to you. The other person can use your public key to verify the signature and make sure the document is authentic and hasn't been tampered with.
Example: Python Code
Here's a simple example using Python to create and verify a digital signature.
# Example of creating and verifying a digital signature in Python
from Crypto.Signature import pkcs1_15
from Crypto.Hash import SHA256
# Data to be signed
message = b'This is my signed document'
# Hash the message
h = SHA256.new(message)
# Sign the hashed message with private key
signature = pkcs1_15.new(private_key).sign(h)
# Verify the signature with public key
try:
pkcs1_15.new(public_key).verify(h, signature)
print("The signature is valid.")
except ValueError:
print("The signature is invalid.")
In this example, the message is hashed and signed with the private_key
. The receiver can use the public_key
to verify that the message is authentic and hasn't been altered.
Secure Communications: Keeping Conversations Private
How It Works
Imagine you want to have a secret conversation with your friend online, but you don't want anyone else to read it. Private-public key cryptography can help! You and your friend each have a public and a private key. You exchange public keys, and then use each other's public keys to encrypt your messages. Even if someone sees the message, they can't unlock it without the corresponding private key. It's like having a secret language only you and your friend understand!
Example: Python Code
Here's a simple example of how to communicate securely using public key encryption:
# Simple example of secure communication using public key encryption in Python
from Crypto.Cipher import PKCS1_OAEP
def encrypt_message(message, public_key):
cipher = PKCS1_OAEP.new(public_key)
return cipher.encrypt(message)
def decrypt_message(ciphertext, private_key):
cipher = PKCS1_OAEP.new(private_key)
return cipher.decrypt(ciphertext)
# User A sends a message to User B
message = b'Hello, this is a secret message.'
# User A encrypts the message with User B's public key
encrypted_msg = encrypt_message(message, public_key)
# User B decrypts the message with their private key
decrypted_msg = decrypt_message(encrypted_msg, private_key)
print(decrypted_msg.decode()) # Output: Hello, this is a secret message.
In this example, User A
encrypts a message using User B's public key
. Only User B
can decrypt it using their private key, ensuring the message remains confidential.
Conclusion
Private-public key cryptography is like a magic shield that protects us online. It's used in lots of ways to keep our information safe, like when we shop online, sign documents, and talk to our friends. It makes our digital lives more secure and allows us to trust the technology we use every day. So the next time you use your credit card online or send an email, remember the power of private-public key cryptography and the invisible shield protecting your information!