The Math Behind ChaCha20: A Deep Dive into its Cryptographic Core
Uncover the secrets of ChaCha20, a blazing fast, secure stream cipher. Learn how it safeguards your data with its unique quarter-round operation and 20 rounds of mixing. Discover why ChaCha20 is a champion in the encryption world, outperforming other methods in speed, security, and simplicity.
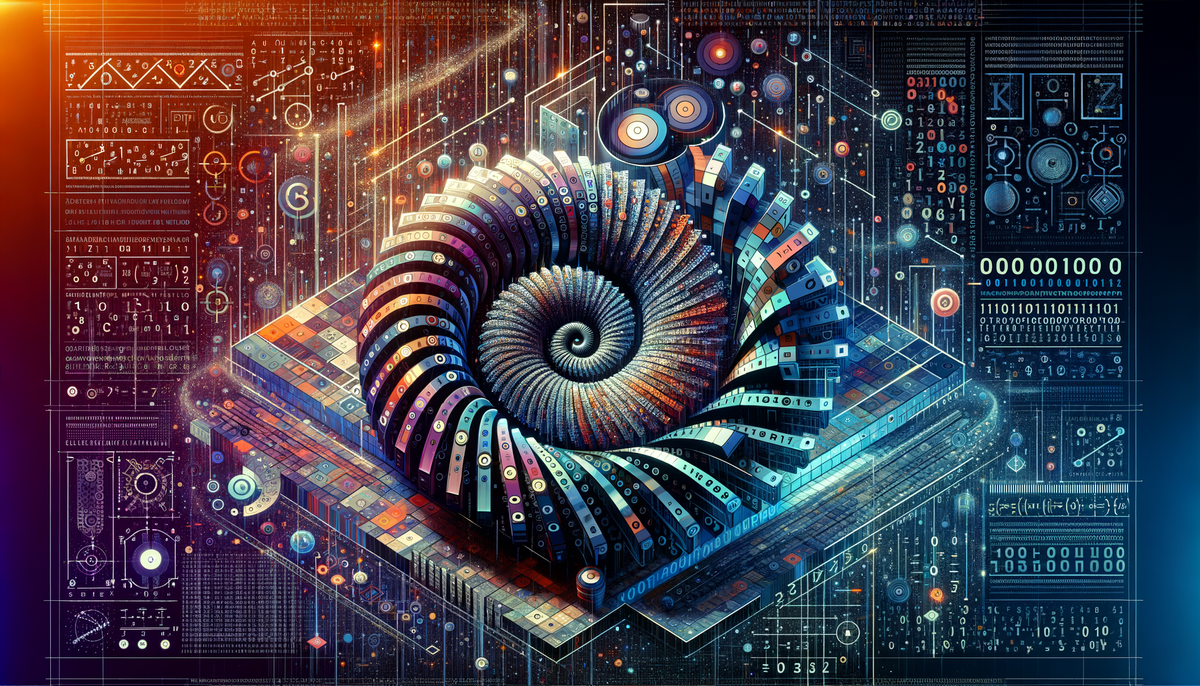
Protecting our data is crucial in today's digital world. Encryption plays a key role in keeping our information secure, and ChaCha20 is a powerful tool for achieving this.
What is ChaCha20 and Why Should You Care?
ChaCha20 is a stream cipher – a type of encryption algorithm that scrambles data bit by bit. It's known for being incredibly fast, robust, and surprisingly easy to understand. Unlike block ciphers which work on chunks of data, ChaCha20 operates on individual bits.
Why is ChaCha20 so popular?
- Speed: It's a speed demon, outperforming many other encryption methods.
- Security: ChaCha20 is highly resistant to various attacks, making it a reliable choice for protecting sensitive data.
- Simplicity: It's designed to be easy to grasp and implement, even for those new to cryptography.
Think of it like a secret code that turns your messages into gibberish only someone with the key can understand. But ChaCha20 does this incredibly fast, making it perfect for applications like secure messaging and web browsing.
Cracking the Code: Unraveling the ChaCha20 Algorithm
At its core, ChaCha20 operates on 512-bit chunks of data. These chunks are organized into a 4x4 matrix, like a digital puzzle grid. Each cell in this matrix holds a 32-bit number. The initial state of the matrix is determined by combining:
- A constant sequence: This is a fixed set of numbers that remains the same for every encryption.
- A key: This is your secret code, the key to unlocking the encryption.
- A nonce: A unique number used for each encryption, ensuring that the same message encrypted multiple times results in different ciphertexts.
- A counter: This is a number that increments with each block of data encrypted, preventing repetition.
Here's a simplified picture of this initial matrix:
(State Vector Example in 4x4 Matrix)
Row 1: constant1 constant2 constant3 constant4
Row 2: key1 key2 key3 key4
Row 3: key5 key6 key7 key8
Row 4: counter nonce1 nonce2 nonce3
The Secret Sauce: The Quarter-Round Operation
The real magic of ChaCha20 lies in the quarter-round operation. It's like a shuffling dance for the numbers in each column of the matrix. Here's how it works:
- Addition: One number is added to another.
- XOR (Exclusive OR): A bitwise operation that compares the bits of two numbers and produces a new number.
- Rotation: The bits of a number are shifted to the left or right.
Let's break it down with a simple example:
Imagine four numbers, A, B, C, and D:
- A = A + B
- D = D ^ A (XOR operation)
- D = Rotate D to the left by 16 bits
- C = C + D
- B = B ^ C
- B = Rotate B to the left by 12 bits
- A = A + B
- D = D ^ A
- D = Rotate D to the left by 8 bits
- C = C + D
- B = B ^ C
- B = Rotate B to the left by 7 bits
These steps are repeated for each column of the matrix, and the process is called a "quarter-round" because it mixes the numbers within a single column.
Here's a code snippet for a quarter-round function in Python:
def quarter_round(a, b, c, d):
a = (a + b) & 0xffffffff
d ^= a
d = (d << 16) | (d >> (32 - 16))
c = (c + d) & 0xffffffff
b ^= c
b = (b << 12) | (b >> (32 - 12))
a = (a + b) & 0xffffffff
d ^= a
d = (d << 8) | (d >> (32 - 8))
c = (c + d) & 0xffffffff
b ^= c
b = (b << 7) | (b >> (32 - 7))
return a, b, c, d
The Grand Dance: Double-Rounds and 20 Rounds of Mixing
ChaCha20 doesn't stop at quarter-rounds. It combines them into "double-rounds" by applying the quarter-round operation across rows and columns of the matrix.
- Column Quarter-Rounds: Apply the quarter-round function to each column of the matrix.
- Diagonal Quarter-Rounds: Apply the quarter-round function to each diagonal of the matrix.
These double-rounds are repeated ten times, resulting in a total of twenty rounds of shuffling and mixing. This thorough mixing process creates a complex, unpredictable output, making it extremely difficult for attackers to crack the encryption.
Why ChaCha20 is a Champion: Unique Features and Advantages
ChaCha20 has some unique features that make it a standout performer:
- Resistant to Timing Attacks: It's designed to take the same amount of time to process any input, preventing attackers from guessing the key by measuring processing times.
- Compatible with Block Ciphers: ChaCha20 can seamlessly replace popular block ciphers like AES in many protocols, offering a modern and efficient alternative.
- Widely Recognized and Proudly Simple: It's gaining popularity and trust in various applications due to its security and ease of use.
A Real-World Example: Putting the Pieces Together
Let's bring this all together with a concrete example of how ChaCha20 encrypts data:
def chacha20_block(key, counter, nonce):
import struct
# The constant - "expand 32-byte k"
constants = [0x61707865, 0x3320646e, 0x79622d32, 0x6b206574]
# Initialize the state matrix
state = constants + list(struct.unpack('<8L', key)) + [counter] + list(struct.unpack('<2L', nonce))
working_state = list(state)
for _ in range(10): # 10 Double rounds
# Column rounds
working_state[0], working_state[4], working_state[8], working_state[12] = quarter_round(working_state[0], working_state[4], working_state[8], working_state[12])
working_state[1], working_state[5], working_state[9], working_state[13] = quarter_round(working_state[1], working_state[5], working_state[9], working_state[13])
working_state[2], working_state[6], working_state[10], working_state[14] = quarter_round(working_state[2], working_state[6], working_state[10], working_state[14])
working_state[3], working_state[7], working_state[11], working_state[15] = quarter_round(working_state[3], working_state[7], working_state[11], working_state[15])
# Diagonal rounds
working_state[0], working_state[5], working_state[10], working_state[15] = quarter_round(working_state[0], working_state[5], working_state[10], working_state[15])
working_state[1], working_state[6], working_state[11], working_state[12] = quarter_round(working_state[1], working_state[6], working_state[11], working_state[12])
working_state[2], working_state[7], working_state[8], working_state[13] = quarter_round(working_state[2], working_state[7], working_state[8], working_state[13])
working_state[3], working_state[4], working_state[9], working_state[14] = quarter_round(working_state[3], working_state[4], working_state[9], working_state[14])
# Add the original state to the working state and serialize
output = [(working_state[i] + state[i]) & 0xffffffff for i in range(16)]
return struct.pack('<16L', *output)
In this code, key
represents your secret key, counter
is a number that increases with each block, and nonce
is a unique value for each encryption. This function takes a block of data, applies the ChaCha20 algorithm, and returns the encrypted output.
Conclusion
Understanding ChaCha20 opens the door to a world of secure data protection. By learning how this algorithm works, you can appreciate its power and efficiency, and even start implementing it in your own projects. Keep exploring the fascinating world of cryptography and stay secure!