Understanding Pointers and Memory Management in GoLang
Dive deep into GoLang's memory management and unravel the secrets of pointers. Discover how pointers work, why they're crucial for efficient programs, and learn about Go's garbage collector. Explore best practices for memory management and avoid common pitfalls like memory leaks.
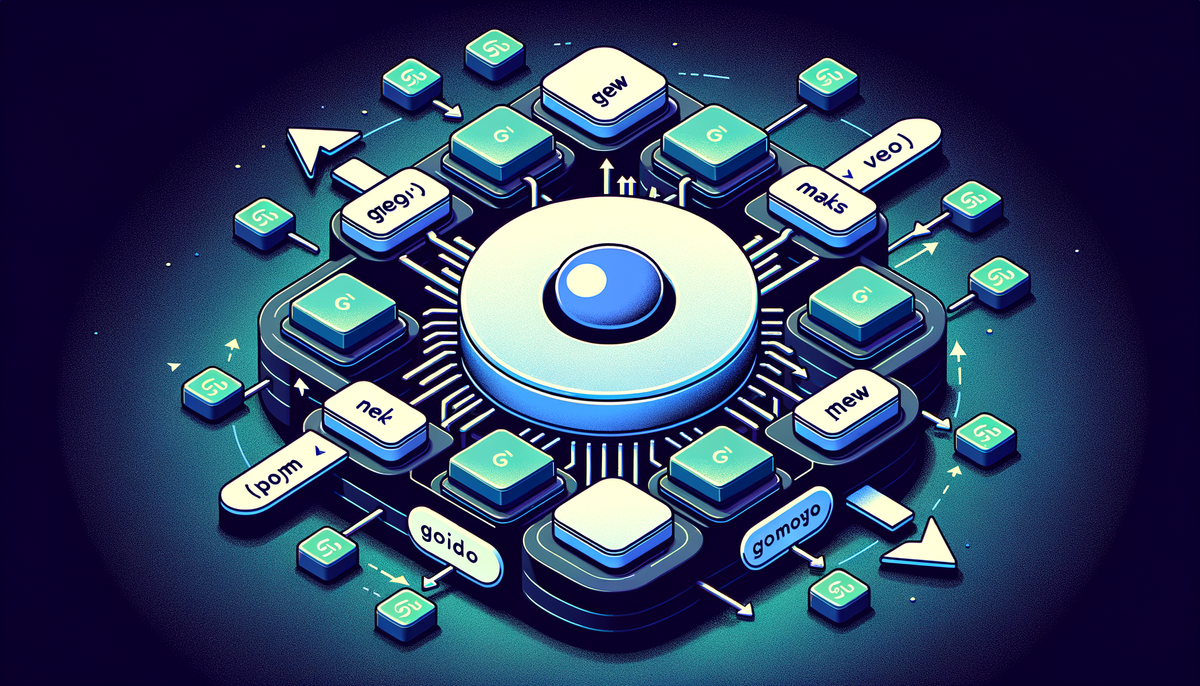
GoLang, often called Go, is known for its simplicity and speed. One of the things that can be hard to understand about Go is how it handles memory, specifically pointers. This article will break down these concepts, making them easier to understand and use in your programs.
What are Pointers?
Imagine you have a box with a toy inside. A pointer is like a special key that tells you where that box is. It doesn't have the toy itself, but it points to the box where the toy lives.
In programming, pointers are variables that store the memory address of other variables. They don't hold the value directly, but they point to the location in the computer's memory where the value is stored. This is super important in Go because it helps us use memory more efficiently and flexibly.
Why Use Pointers?
- Faster Programs: Pointers can make programs run faster because they don't have to copy big pieces of data. Imagine you have a really heavy box, and you want to show it to your friends. It's easier to just tell them where the box is than to carry it around everywhere.
- More Control: Pointers give you more control over how your program interacts with memory. You can change the values of variables without having to move the whole data around.
How Pointers Work in Go
Creating Pointers
To make a pointer, you use the *
symbol followed by the type of the variable you want the pointer to point to.
var ptr *int
This creates a pointer named ptr
that can point to an integer.
Using Pointers
Let's look at an example to see how pointers work.
package main
import "fmt"
func main() {
var a int = 42 // Create an integer variable named `a`
var p *int // Create a pointer to an integer named `p`
p = &a // Store the address of `a` in the pointer `p`
fmt.Println("Value of a:", a) // This prints: 42
fmt.Println("Address of a:", p) // This prints the memory location of `a`
fmt.Println("Value at address p:", *p) // This prints: 42
}
Explanation
- We make an integer called
a
and give it the value42
. - We make a pointer to an integer called
p
. - We use the
&
symbol to get the address ofa
and store it in the pointerp
. - Now
p
knows wherea
lives in memory. - We use the
*
symbol to get the value thatp
is pointing to, which is42
.
Changing Values Through Pointers
One cool thing about pointers is that you can use them to change the values of variables they point to.
package main
import "fmt"
func main() {
x := 58
ptr := &x
fmt.Println("Before:", x) // Output: 58
*ptr = 100
fmt.Println("After:", x) // Output: 100
}
Explanation
- We make an integer named
x
and set its value to58
. - We make a pointer
ptr
that points tox
. - We use
*ptr
to access the value ofx
and change it to100
. Sinceptr
is pointing tox
, changing the value throughptr
actually changes the value ofx
.
Example: Swapping Values with Pointers
Let's see how to swap two values using pointers.
package main
import "fmt"
func swap(a, b *int) {
temp := *a // Store the value of `a` in a temporary variable
*a = *b // Put the value of `b` into `a`
*b = temp // Put the original value of `a` into `b`
}
func main() {
num1 := 5
num2 := 10
fmt.Println("Before swap:", num1, num2)
swap(&num1, &num2)
fmt.Println("After swap:", num1, num2)
}
Explanation
- We create a function called
swap
that takes two pointers to integers as input. - Inside
swap
, we use a temporary variable to hold the original value ofa
. - Then we swap the values of
a
andb
by using the pointers. - In the
main
function, we create two integers and then callswap
to switch their values.
Memory Management in Go
Memory management is how the computer keeps track of where data is stored and makes sure it doesn't run out of space. Go has a built-in system called a "garbage collector" that does this automatically. But understanding how memory works can help you write better Go programs.
Getting Memory
Go has two ways to get memory:
new
: This gives you a pointer to a chunk of memory, but it doesn't put any value in it yet.
p := new(int)
fmt.Println(*p) // Output: 0 (default value of int)
make
: This is for making special data structures like slices (lists), maps (dictionaries), and channels (communication channels). It allocates memory and sets up the structure.
s := make([]int, 5)
fmt.Println(s) // Output: [0 0 0 0 0]
Example: Using make
and new
package main
import "fmt"
func main() {
// Using new
p := new(int)
fmt.Println(*p) // Output: 0 (default int)
*p = 45
fmt.Println(*p) // Output: 45
// Using make
s := make([]int, 3)
fmt.Println(s) // Output: [0 0 0]
s[0] = 1
s[1] = 2
s[2] = 3
fmt.Println(s) // Output: [1 2 3]
}
Good Practices for Memory in Go
Avoiding Memory Leaks
- Clean Up: Always try to release the memory your program isn't using anymore. Even though Go has a garbage collector, it's good practice to do your part.
- Use Tools: Go has tools like
pprof
that can help you find places where your program might be using too much memory or doing things inefficiently.
Example: Using pprof
package main
import (
"fmt"
"net/http"
_ "net/http/pprof"
)
func main() {
go func() {
fmt.Println(http.ListenAndServe("localhost:6060", nil))
}()
// Your application logic here
}
This code starts a server on localhost:6060
that you can use to analyze how your program is using memory.
Debugging Memory Problems
Finding memory leaks or performance issues can be tricky. pprof
is really helpful, but it's also good to know about common mistakes.
Example: Common Memory Mistake
package main
func main() {
data := make([]byte, 0, 100000) // Create a slice with initial capacity
for i := 0; i < 100000; i++ {
data = append(data, byte(i)) // Keep appending to the slice
}
}
Explanation
This code makes a slice that can hold 100,000 bytes. The problem is that we keep adding more bytes to the slice in a loop, which can use up a lot of memory. Go has to keep getting more space to hold the growing slice.
Conclusion
Knowing about pointers and memory management in Go is super important for making programs that are fast and use memory wisely. By understanding how to use pointers to access memory directly and how Go handles memory with its new
, make
, and garbage collector, you can write efficient and reliable Go programs.
Happy coding!