Using Loops and Conditions in Bash Scripts: Structure Your Scripts for Complex Tasks
Master Bash scripting by leveraging loops and conditions for powerful automation. Learn how to repeat actions, make decisions, and structure scripts for complex tasks with examples for file manipulation, network checks, and more.
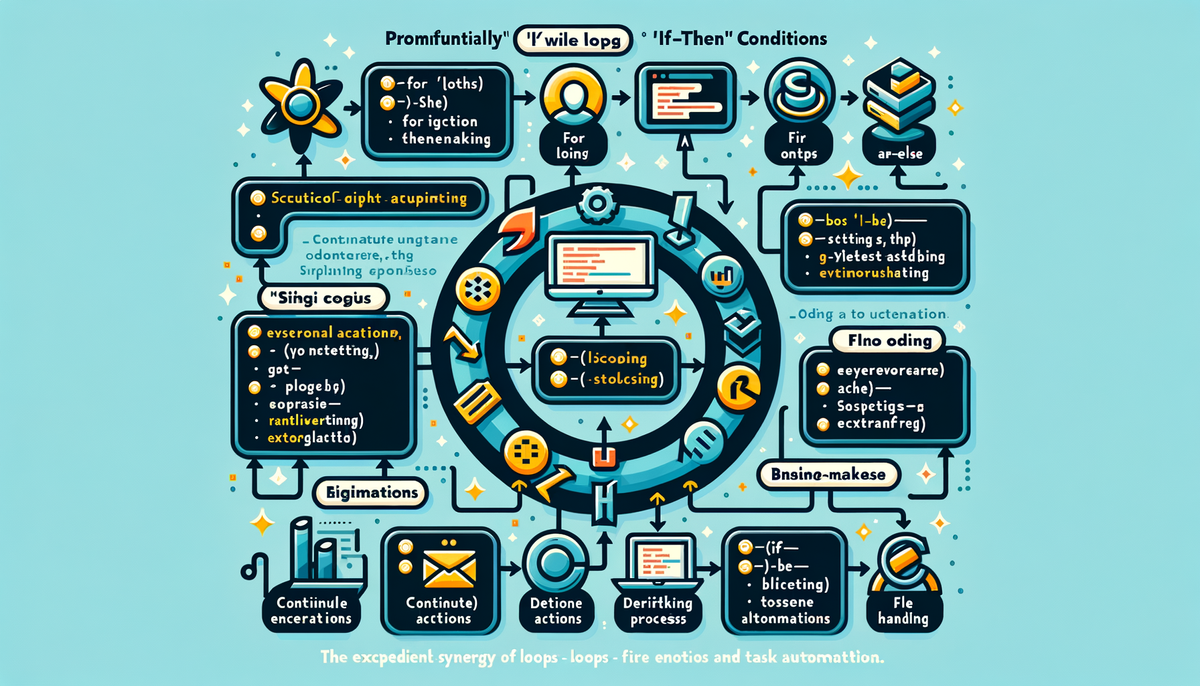
Bash scripting is a powerful tool for automating tasks, managing systems, and making your life easier. One of the key features of Bash scripting is its ability to use loops and conditions. These features allow you to write scripts that can repeat tasks, make decisions, and adapt to different situations. In this article, we'll explore how to use loops and conditions in Bash scripts, and you'll learn how to structure your scripts for complex tasks.
Understanding Loops and Conditions
Let's imagine you're cleaning your room. You might need to repeat the same action, like picking up toys, several times. That's what a loop does in a script. It helps you repeat commands without writing them again and again.
Now, imagine you need to decide whether to wear a jacket or not based on the weather. That's what a condition does. It allows your script to make choices based on certain rules.
Loops in Bash: Repeating Actions
1. for
Loops: Repeating for a Fixed Number of Times
Imagine you want to send a message to each of your five friends. You could use a for
loop to send the message five times.
Basic Syntax:
for VAR in LIST; do
COMMANDS
done
Example: Printing Numbers 1 to 5
#!/bin/bash
for i in {1..5}; do
echo "Number: $i"
done
Explanation:
for i in {1..5}
: This loop will run five times, with the variablei
taking on the values 1, 2, 3, 4, and 5.echo "Number: $i"
: Inside the loop, theecho
command prints the current value ofi
.
This code will output:
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
Example: Iterating Over Files in a Directory
#!/bin/bash
for file in /path/to/directory/*; do
echo "Processing file: $file"
done
Explanation:
for file in /path/to/directory/*
: This loop goes through each file in the specified directory.echo "Processing file: $file"
: For each file, it prints a message indicating the file being processed.
2. while
Loops: Repeating Until a Condition is Met
Imagine you want to keep asking for a number until the user enters a number greater than 10. You could use a while
loop for this.
Basic Syntax:
while CONDITION; do
COMMANDS
done
Example: Counting Down from 5 to 1
#!/bin/bash
i=5
while [ $i -gt 0 ]; do
echo "Countdown: $i"
i=$((i - 1))
done
Explanation:
i=5
: This sets the initial value of the counteri
to 5.while [ $i -gt 0 ]; do
: This loop will continue as long as the value ofi
is greater than 0.echo "Countdown: $i"
: Inside the loop, it prints the current value ofi
.i=$((i - 1))
: This line subtracts 1 from the value ofi
in each iteration.
This code will output:
Countdown: 5
Countdown: 4
Countdown: 3
Countdown: 2
Countdown: 1
Example: Reading a File Line by Line
#!/bin/bash
file="example.txt"
while read -r line; do
echo "Line: $line"
done < "$file"
Explanation:
file="example.txt"
: This sets the filename toexample.txt
.while read -r line
: This loop reads each line from the file.echo "Line: $line"
: It prints each line read from the file.
Conditions in Bash: Making Decisions
Imagine you're playing a game where you need to check if you've won or lost. You could use an if-then-else
statement to decide what to do based on the outcome of the game.
Basic Syntax:
if CONDITION; then
COMMANDS
elif ANOTHER_CONDITION; then
COMMANDS
else
COMMANDS
fi
Example: Checking if a Number is Positive, Negative, or Zero
#!/bin/bash
num=-3
if [ $num -gt 0 ]; then
echo "$num is positive"
elif [ $num -lt 0 ]; then
echo "$num is negative"
else
echo "$num is zero"
fi
Explanation:
if [ $num -gt 0 ]; then
: This checks if the value ofnum
is greater than 0.elif [ $num -lt 0 ]; then
: If the previous condition is false, this checks ifnum
is less than 0.else
: If both previous conditions are false, this part is executed.
This code will output:
-3 is negative
Example: Checking for File Existence
#!/bin/bash
file="example.txt"
if [ -e "$file" ]; then
echo "$file exists"
else
echo "$file does not exist"
fi
Explanation:
file="example.txt"
: This sets the filename toexample.txt
.if [ -e "$file" ]; then
: This checks if the file exists.
Combining Loops and Conditions: Powerful Combinations
You can combine loops and conditions to create complex and useful scripts.
Example: Checking File Sizes in a Directory
#!/bin/bash
for file in /path/to/directory/*; do
if [ -f "$file" ]; then
size=$(stat -c%s "$file")
if [ $size -gt 1000000 ]; then
echo "Large file: $file"
else
echo "Small file: $file"
fi
fi
done
Explanation:
for file in /path/to/directory/*
: This loops through all files in the specified directory.if [ -f "$file" ]; then
: This checks if the current item is a regular file.size=$(stat -c%s "$file")
: This gets the size of the file in bytes.if [ $size -gt 1000000 ]; then
: This checks if the file size is greater than 1MB.
This script will print messages indicating whether each file is large or small.
Example: Pinging Multiple Hosts
#!/bin/bash
hosts=("google.com" "github.com" "nonexistent.host")
for host in "${hosts[@]}"; do
if ping -c 1 "$host" &> /dev/null; then
echo "$host is reachable"
else
echo "$host is unreachable"
fi
done
Explanation:
hosts=("google.com" "github.com" "nonexistent.host")
: This defines an array of hostnames.for host in "${hosts[@]}"; do
: This loop iterates through each host in the array.if ping -c 1 "$host" &> /dev/null; then
: This checks if the host can be pinged successfully.else
: This part is executed if the host is unreachable.
This script will try to ping each host and print whether it's reachable or not.
Conclusion
By understanding and using loops and conditions in your Bash scripts, you gain the ability to automate complex tasks, manage systems more effectively, and streamline your work. Experiment with the examples provided, and you'll soon become a proficient Bash scripter. Happy scripting!