What is a Private-Public Key Pair? - A Comprehensive Explanation
Secure your digital life with private-public key pairs! Learn how this powerful encryption method protects your data and allows secure communication. Discover real-world uses, including secure websites, email encryption, and blockchain technology. Get a comprehensive explanation with code examples!
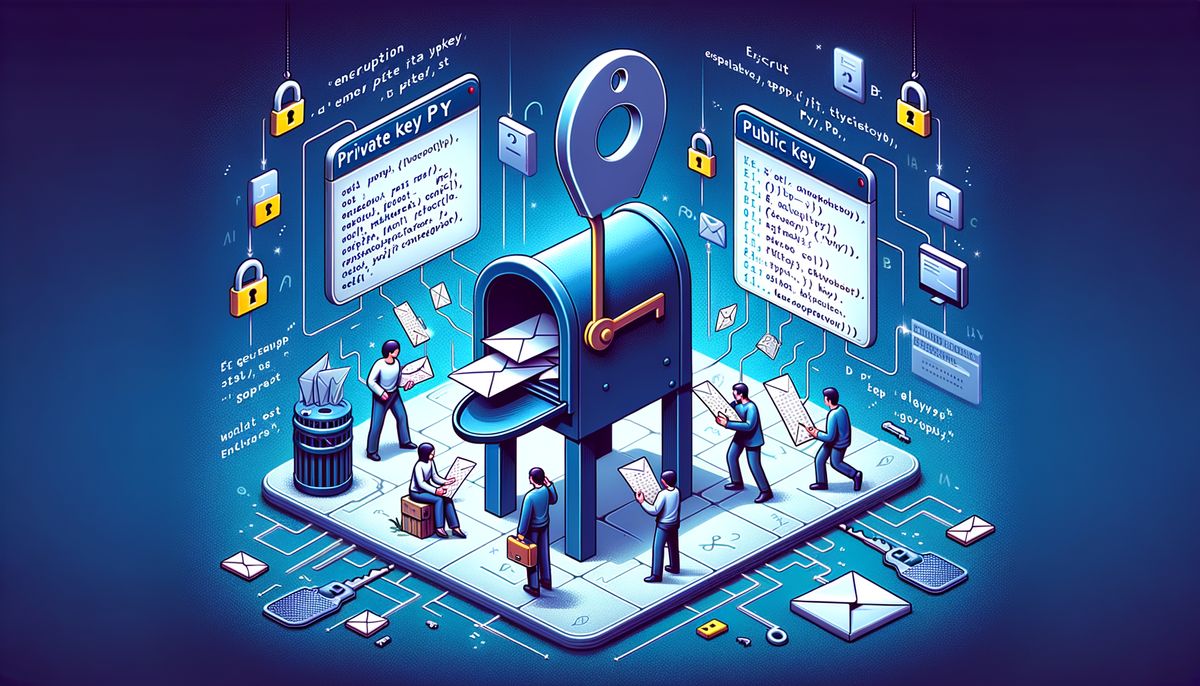
In today's digital world, we share a lot of information online. It's important to keep this information safe from hackers. One way to do this is with a private-public key pair. This is like a special lock and key system for your digital information.
What is a Private-Public Key Pair?
Imagine you have a mailbox. You give everyone your mailbox address (the public key) so they can send you letters. But, you keep the key to your mailbox (the private key) secret. Only you can open the mailbox and read the letters.
A private-public key pair is similar. It's a set of two special codes that work together. One code (the public key) is shared with everyone. The other code (the private key) is kept secret. These codes are used to encrypt and decrypt information, making it safe from anyone who doesn't have the private key.
Why Are Private-Public Key Pairs Important?
Private-public key pairs help keep your information safe because:
- Only you can decrypt information encrypted with your public key: Just like only you can open your mailbox, only you can unlock information that has been locked with your public key.
- They make it hard for hackers to steal your information: Hackers can't easily unlock information that's encrypted with a private-public key pair unless they have the private key.
Parts of a Private-Public Key Pair
- Public Key: Think of this like your email address. You can share it with anyone. This key is used to encrypt messages so only the person with the matching private key can read them.
- Private Key: Think of this like the password to your email. You keep it secret and safe. This key is used to decrypt messages that were encrypted with the matching public key.
How Do Private-Public Key Pairs Work?
Let's imagine you want to send a secret message to your friend. You can use a private-public key pair to make sure only your friend can read the message.
Sending a Secret Message
- Your friend creates a key pair: Your friend generates a public and private key. They share their public key with you.
- You encrypt your message: You use your friend's public key to encrypt your message. This makes the message unreadable to anyone else.
- You send the encrypted message: You send the encrypted message to your friend.
- Your friend decrypts the message: Your friend uses their private key to decrypt the message. Now they can read your secret message!
An Example with Code
We can use Python code to see how this works. First, we need to install a library called cryptography
.
pip install cryptography
Now, let's create a private-public key pair.
from cryptography.hazmat.primitives.asymmetric import rsa
from cryptography.hazmat.primitives import serialization
# Generate private key
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048,
)
# Generate public key
public_key = private_key.public_key()
# Save the private key
with open("private_key.pem", "wb") as f:
f.write(
private_key.private_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PrivateFormat.TraditionalOpenSSL,
encryption_algorithm=serialization.NoEncryption(),
)
)
# Save the public key
with open("public_key.pem", "wb") as f:
f.write(
public_key.public_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PublicFormat.SubjectPublicKeyInfo,
)
)
Encrypting and Decrypting a Message
Now let's see how to encrypt and decrypt a message.
from cryptography.hazmat.primitives.asymmetric import padding
from cryptography.hazmat.primitives import hashes
# Encrypt message using public key
message = b'Secret message'
ciphertext = public_key.encrypt(
message,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
# Decrypt message using private key
decrypted_message = private_key.decrypt(
ciphertext,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
print(decrypted_message.decode('utf-8'))
This example shows how you can securely send messages using a private-public key pair.
Real-World Uses
Private-public key pairs are used in many different ways to keep information safe.
- Secure websites: When you visit a secure website (like an online bank), your browser uses a private-public key pair to communicate with the website safely.
- Email encryption: Some email providers use private-public key pairs to encrypt your emails, making them unreadable to anyone except the intended recipient.
- Blockchain: Cryptocurrencies like Bitcoin use private-public key pairs to verify transactions.
Solving Your Problems
- Problem: You want to keep your online information safe.
- Solution: Using private-public key pairs helps ensure only you can access your information.
- Problem: You want to send a secret message to a friend.
- Solution: You can encrypt the message with your friend's public key, ensuring only they can decrypt it.
By using private-public key pairs, you can help keep your digital life more secure. They are a powerful tool for protecting your online information.